C Programming Syntax – A Complete Guide to Mastering C Language Syntax
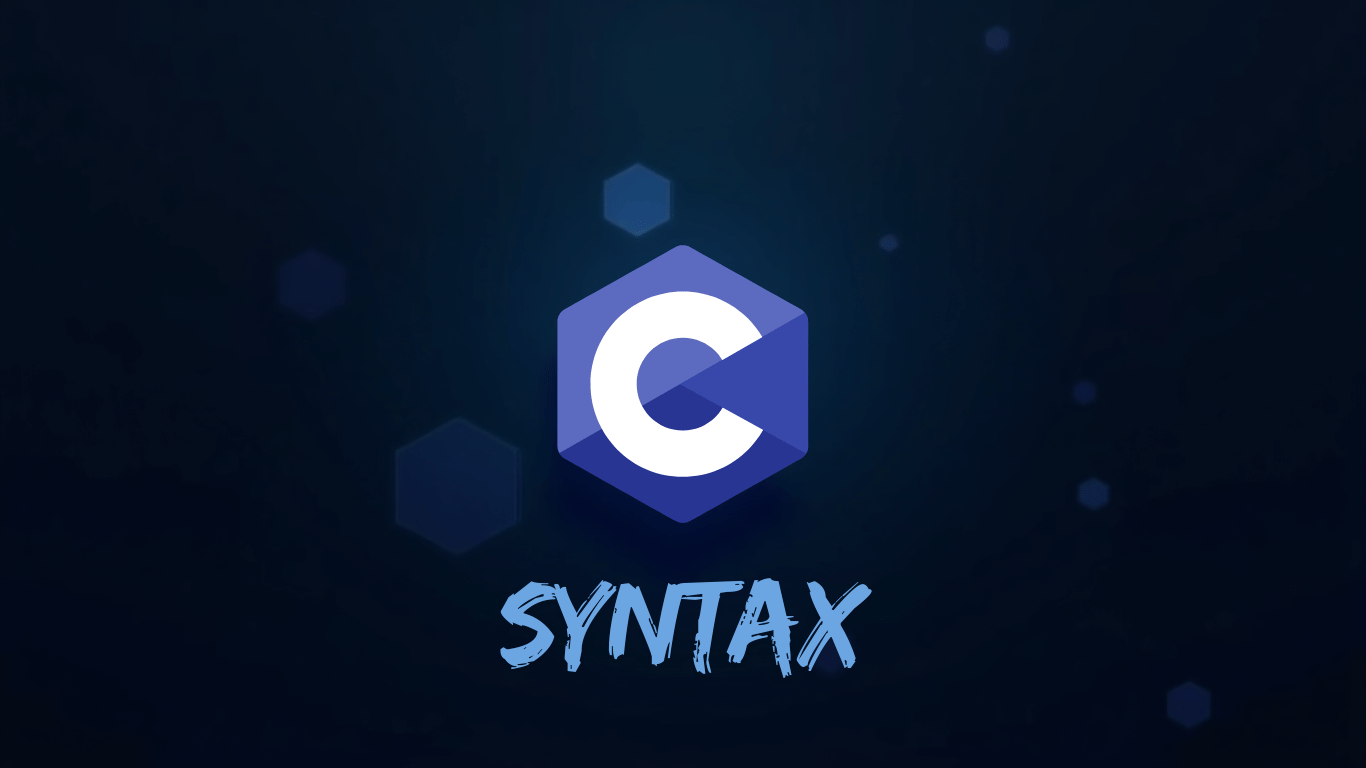
Introduction: Understanding C Programming Syntax – The Key to Efficient Coding
Have you ever wanted to learn a programming language that gives you complete control over a computer’s hardware and system operations? C is one of the most powerful programming languages, widely used in software development, operating systems, and embedded systems. But to harness its full potential, you must first understand its syntax.
Mastering syntax isn’t just about memorizing rules; it’s about learning how to communicate with the computer in a structured way. Whether you’re new to coding or transitioning from another language, this guide will help you grasp the fundamentals of C programming syntax.
Table of Contents
What is C Programming Syntax?
Definition of Syntax in C
C programming syntax defines the structure and rules that dictate how C programs are written and compiled. Unlike interpreted languages, C is compiled, meaning even minor syntax errors can prevent the program from running.
Key Components of C Syntax
Component | Description | Example |
---|---|---|
Keywords | Reserved words in C | int, return, if, while |
Identifiers | Names for variables & functions | int age; |
Operators | Used for arithmetic & logic | +, -, *, /, % |
Statements | Executable lines of code | printf("Hello"); |
Control Flow | Structures for decision-making | if, switch, loops |
Understanding these basic elements lays the foundation for writing effective C programs.
Basic Structure of a C Program
A C program follows a well-defined structure. Here’s a simple example:
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Explanation of Key Components
- Preprocessor Directives (
#include
): These include necessary libraries, such asstdio.h
for input and output functions. - Main Function (
int main()
): Every C program must have amain()
function, which serves as the entry point. - Statements (
printf()
,return 0;
): Executable commands that define program behavior.
Fundamental C Syntax Elements
Variables and Data Types
Variables store data, while data types define what kind of data a variable holds.
Common C data types:
Data Type | Description | Example |
---|---|---|
int | Stores integers | int age = 25; |
float | Stores decimals | float pi = 3.14; |
char | Stores single characters | char grade = 'A'; |
double | Stores large floating-point numbers | double gpa = 3.75; |
Operators in C
- Arithmetic Operators:
+, -, *, /, %
- Comparison Operators:
==, !=, >, <, >=, <=
- Logical Operators:
&&, ||, !
Example:
if (age >= 18 && age < 65) {
printf("You are eligible to work.");
}
Control Flow Statements in C
Conditional Statements (If-Else, Switch Case Syntax in C)
If-Else Syntax in C
if (age >= 18) {
printf("Adult");
} else {
printf("Minor");
}
Switch Case Syntax in C
switch(choice) {
case 1:
printf("Option 1 selected.");
break;
case 2:
printf("Option 2 selected.");
break;
default:
printf("Invalid choice.");
}
When deciding between if-else
and switch
, use if-else
for range-based conditions and switch
for multiple discrete values.
Looping Constructs in C
While Loop Syntax in C
A while
loop executes as long as the condition remains true.
int i = 0;
while (i < 5) {
printf("%d ", i);
i++;
}
For Loop Syntax in C
Best used when the number of iterations is known.
for (int i = 0; i < 5; i++) {
printf("%d ", i);
}
Loop Type | Use Case | Example |
---|---|---|
while | Runs while condition is true | while(i < 5) { i++; } |
for | Known iteration count | for(i=0; i<5; i++) |
do-while | Runs at least once | do { ... } while (condition); |
Functions in C
Syntax of a Function in C
Functions allow code reusability and modularity.
int add(int a, int b) {
return a + b;
}
- Function Definition: Defines what the function does.
- Function Call: Executes the function.
- Return Type: Determines the data type of the returned value.
Common Errors and Debugging in C Syntax
Common Syntax Mistakes
- Missing semicolons (
;
) at the end of statements. - Mismatched brackets
{}
in loops and conditions. - Incorrect data type assignments.
- Using undeclared variables.
Debugging Tips
- Use
printf()
to check variable values. - Enable compiler warnings for catching errors early.
- Use debugging tools like GDB (GNU Debugger).
Frequently Asked Questions (FAQ) on C Programming Syntax
What is C programming syntax?
C syntax refers to the set of rules that define how programs are written and structured in the C language.
What is the while loop syntax in C?
The while
loop in C executes code repeatedly while a condition is true:
while(condition) {
// code block
}
How does switch case syntax in C work?
A switch
statement evaluates an expression and executes the matching case block:
switch(expression) {
case value1:
// Code
break;
case value2:
// Code
break;
default:
// Code
}
What are the main components of C syntax?
C syntax includes keywords, variables, operators, control statements, loops, and functions.
Conclusion: Mastering C Syntax for Efficient Programming
Mastering C syntax is your first step toward writing efficient and bug-free programs. By understanding variables, loops, functions, and control structures, you gain the ability to solve complex problems effectively.
Are you ready to start coding? Open your compiler, write a simple C program, and test your newfound knowledge today!