Mastering Python Numbers: The Ultimate Guide in 2025
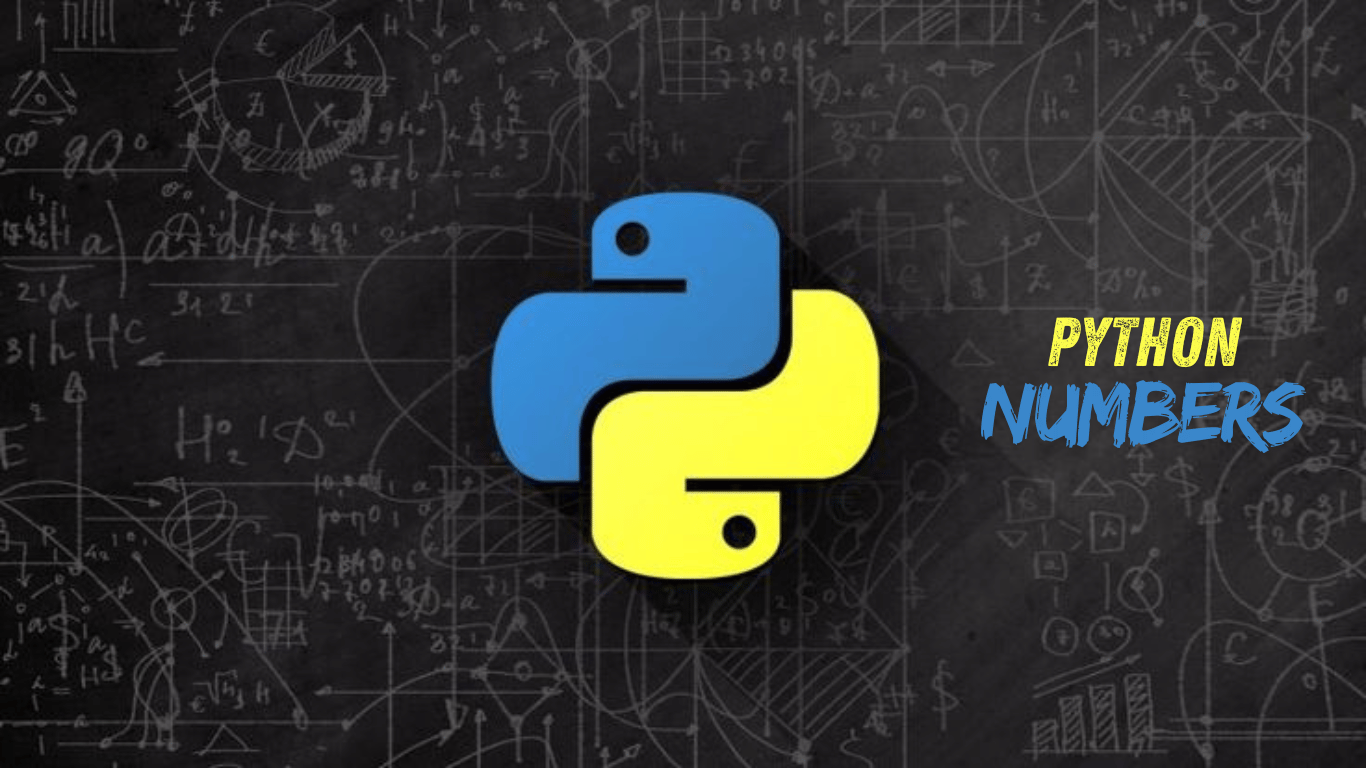
Welcome to the whimsical and ever-exciting world of Python numbers, where digits swirl around like an intricate dance, decimals sparkle with grace, and complex numbers—well, they sometimes get a little too complicated for their own good! As we take a stroll through this mathematical landscape, we’ll explore the fundamental number types in Python: integers, floats, and complex numbers. But don’t worry—this guide won’t be all work and no play. We’re going to sprinkle in some humor, quirky examples, and maybe even a few jokes to keep things lively, fun, and, most importantly, easy to understand.
In the realm of Python programming, numbers are the heart of everything. They allow you to perform calculations, measure things, and keep track of data. Whether you’re simply counting items in a list, calculating the price of a pizza, or doing complex scientific simulations, numbers are at the core of your code. But not all numbers are created equal. There are distinct types, and each has its own role to play in your coding adventures.
First, let’s talk about the most straightforward type: integers. These are the whole numbers, the simple yet reliable workhorses of the number world. If you need to represent quantities, counts, or indexes, integers are your go-to guys. They’re whole, they’re precise, and they’re always ready to step up to the plate when it’s time for simple math.
Whether you’re just starting to learn Python or you’ve been coding for years, mastering numbers is an essential skill. By the end of this guide, you’ll have a solid understanding of how to work with integers, floats, and complex numbers, and you’ll be able to use them effectively in your own projects. So, let’s embark on this journey through the fascinating world of Python numbers—where digits come alive, decimals shine, and complex numbers add that special touch of intrigue! Let the fun begin!
Table of Contents
Python Numbers : The Basics :
Python numbers are the building blocks of arithmetic and calculations in programming. They come in three primary flavors:
- Integers (int) : Whole numbers without any fractional or decimal component.
- Floating-Point Numbers (float) : Numbers with decimal points, perfect for precise calculations.
- Complex Numbers (complex) : Numbers that include a real and imaginary part, used in advanced computations.
Let’s meet our numerical heroes and see how they work with a touch of humor.
Python Numbers – The Integer Extravaganza :
Integers are the no-nonsense characters of the number world. They’re simple, straightforward, and always ready to perform basic arithmetic. Imagine them as the dependable workhorses of your calculations.
Example :
# Integer Adventures
def integer_fun():
hero_age = 25
sidekick_age = 7
print(f"Our hero is {hero_age} years old.")
print(f"The sidekick is {sidekick_age} years old.")
print(f"Together , their combined age is {hero_age + sidekick_age}.")
#Run the integer fun
integer_fun()
In this example, hero_age and sidekick_age are both integers. The script performs a simple addition to show their combined age. It’s basic math, but always reliable!
Python Numbers – The Float Fiesta :
Floats bring a bit of flair to your calculations with their decimal points. They’re like the sophisticated artists of the number world, adding precision and detail to your data.
Example :
# Float Follies
def float_fun():
pi_value = 3.14159
e_value = 2.71828
print(f"The value of Pi is approximately {pi_value}")
print(f"The value of e is approximately {e_value}")
print(f"Adding them gives us : {pi_value+e_value}")
# Run the float fun
float_fun()
Complex numbers are like the eccentric geniuses of the number world. They have both a real and imaginary part, and they’re essential for advanced mathematics and engineering.
Example :
# Complex Capers
def complex_fun():
complex_number = 2 + 3j
print(f"Our complex number is {complex_number}.")
print(f"The real part is {complex_number.real}.")
print(f"The imaginary part is {complex_number.imag}.")
print(f"The conjugate of our complex number is {complex_number.conjugate()}.")
# Run the complex fun
complex_fun()
In this script, complex_number represents a complex number with a real part of 2 and an imaginary part of 3. The script demonstrates how to access the real part, imaginary part, and conjugate. It’s complex, but with a bit of humor!
The Power of Python’s Number Operations :
Python Numbers come with a suite of operations that make them incredibly versatile. From basic arithmetic to advanced calculations, Python numbers handle it all.
Example :
# Number Operations Fun
def number_operations():
a = 8
b = 3.5
c = 1 + 2j
print(f"Addition: {a} + {b} = {a + b}")
print(f"Subtraction: {a} - {b} = {a - b}")
print(f"Multiplication: {a} * {b} = {a * b}")
print(f"Division: {a} / {b} = {a / b}")
print(f"Complex Multiplication: {a} * {c} = {a * c}")
# Run the number operations
number_operations()
This script shows basic arithmetic operations with integers and floats, as well as multiplication with complex numbers. Python’s number operations are straightforward yet powerful, handling everything from simple math to more advanced calculations.
Fun Facts About Python Numbers :
- Integers are Infinite : Python’s integers can grow as large as your machine’s memory allows. There’s practically no limit to how big an integer can be.
- Floating-Point Precision : Floats are precise but not perfect. They can sometimes lead to small rounding errors due to the way computers handle decimal points.
- Complex Numbers in Real Life : Complex numbers are not just for mathematicians—they’re used in electrical engineering, quantum physics, and signal processing.
Python numbers are versatile tools that make programming both powerful and fun. Whether you’re working with the straightforward integers, the elegant floats, or navigating the complex complexities of complex numbers, Python handles them all with grace and efficiency.
This ultimate guide to Python numbers has explored their key features in a way that’s easy to understand, spiced up with humor and simplicity. By embracing the unique characteristics of integers, floats, and complex numbers, you’ll unlock the true power of Python in your projects. So, the next time you dive into Python coding, remember these quirky number types and how they can make your coding experience not only effective but also enjoyable!