C Programming Language – A Beginner’s Guide to Learn C Programming
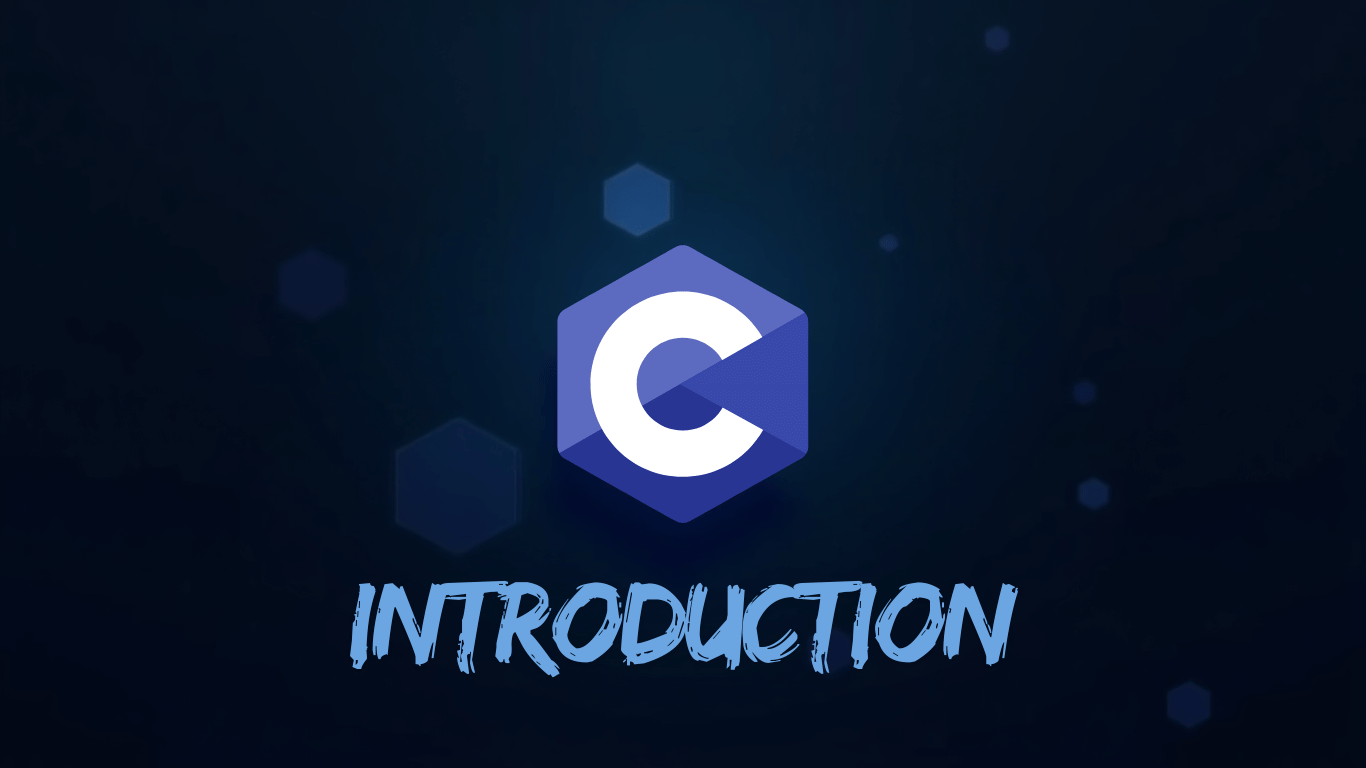
Introduction: Why Learn C Programming Language?
Have you ever wanted to understand how software truly works? Imagine having the power to write code that interacts directly with your computer’s hardware, build efficient applications, and grasp the fundamentals that form the backbone of modern programming languages. That’s exactly what learning C programming can offer you.
C isn’t just another programming language; it’s the foundation upon which many others, like Python, Java, and C++, are built. By mastering C, you develop problem-solving skills that translate to nearly every aspect of software development. Whether you want to code an operating system, build embedded systems, or optimize performance-critical applications, C is where it all begins.
If you’re ready to embark on this journey, let’s dive into what makes C so powerful and why it remains one of the most relevant programming languages today.
Table of Contents
What is the C Programming Language?
C is a general-purpose, procedural programming language that was first introduced in 1972 by Dennis Ritchie at Bell Labs. Originally designed for system programming, it became widely used for developing operating systems, including the early versions of UNIX.
Key Features of C Programming
- Low-Level Access – Unlike high-level languages, C gives you direct control over hardware.
- Portability – Write once, compile anywhere.
- Efficiency & Speed – Optimized for performance-critical applications.
- Rich Standard Library – Provides built-in functions for handling data, I/O, memory management, and more.
- Versatility – Used in system programming, embedded systems, game development, and more.
If you’re aiming for a solid programming foundation, C is the best place to start.
Why Should You Learn C Programming?
Here are some compelling reasons why learning C can be a game-changer for your coding journey:
- Build a Strong Foundation – Many modern languages, such as Java, C++, and Python, borrow concepts from C.
- Develop Efficient Code – C enables memory management and fine-tuned performance optimizations.
- Career Opportunities – Many industries, including embedded systems, operating systems, and game development, require C proficiency.
- Understand How Computers Work – Learning C gives insight into how operating systems and hardware interact.
Setting Up a C Programming Compiler
Before you write your first C program, you need a compiler. A compiler translates your human-readable C code into machine code that the computer understands.
What is a C Compiler?
A C compiler takes the C source code and converts it into an executable file. Popular compilers include:
Compiler | Platform |
---|---|
GCC (GNU Compiler Collection) | Windows, macOS, Linux |
Turbo C | Windows |
Clang | macOS, Linux |
Microsoft Visual C++ Compiler | Windows |
Installing a C Compiler
Here’s how to install a compiler based on your operating system:
- Windows: Install MinGW or Dev-C++.
- macOS: Install Xcode Command Line Tools.
- Linux: Use
sudo apt install gcc
to install GCC. - Online: Try an online compiler like Ideone or OnlineGDB.
Basic Syntax and First C Program
Once your compiler is set up, it’s time to write your first program.
Structure of a C Program
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
Understanding the Syntax
#include <stdio.h>
– Includes the standard I/O library.int main()
– The entry point of a C program.printf()
– Prints text to the console.return 0;
– Indicates successful program termination.
Key Concepts in C
Concept | Description | Example |
---|---|---|
Variables | Store data | int num = 10; |
Data Types | Define variable type | int, float, char |
Operators | Perform calculations | +, -, *, /, % |
Control Structures | Manage program flow | if, else, switch |
Loops | Execute repeatedly | for, while, do-while |
Common Mistakes Beginners Make in C Programming
- Forgetting to include header files (
#include <stdio.h>
). - Using the wrong data type (e.g., assigning a float to an int without casting).
- Ignoring memory allocation issues (e.g., not freeing allocated memory).
- Forgetting to use
{}
in conditional statements.
Avoid these mistakes early on to become a proficient C programmer.
Advanced Topics to Explore After Learning Basics
Pointers in C
- Understand memory addresses and direct access to variables.
- Example:
int *ptr; ptr = #
File Handling in C
- Read and write files using
fopen()
,fprintf()
,fscanf()
.
Data Structures in C
- Learn about arrays, linked lists, stacks, queues, trees, and graphs.
Frequently Asked Questions (FAQ) on C Programming Language
What is the best way to learn C programming?
Start with the basics, practice daily, and work on small projects to reinforce concepts.
Which C programming compiler is best for beginners?
GCC is the best choice for cross-platform development. Dev-C++ is also beginner-friendly for Windows users.
Is C programming still relevant today?
Absolutely! C remains widely used in system programming, embedded systems, and high-performance applications.
How long does it take to learn C programming?
- Basics: 2-4 weeks
- Intermediate level: 2-3 months
- Advanced concepts: 6+ months with real-world projects
Conclusion: Your Journey in C Programming Begins Now!
Learning C is more than just writing code; it’s about understanding the building blocks of modern software. Whether you’re a beginner looking to break into programming or an experienced developer wanting to refine your skills, C provides the perfect starting point.
Are you ready to dive deeper? Install a compiler, write your first program, and start experimenting with C today. The more you practice, the more confident you’ll become. Happy coding!
By following this guide, you’re setting yourself up for success in C programming. If you found this article helpful, share it with others who are starting their coding journey!