Introduction to JavaScript: Your Gateway to the Web’s Magic
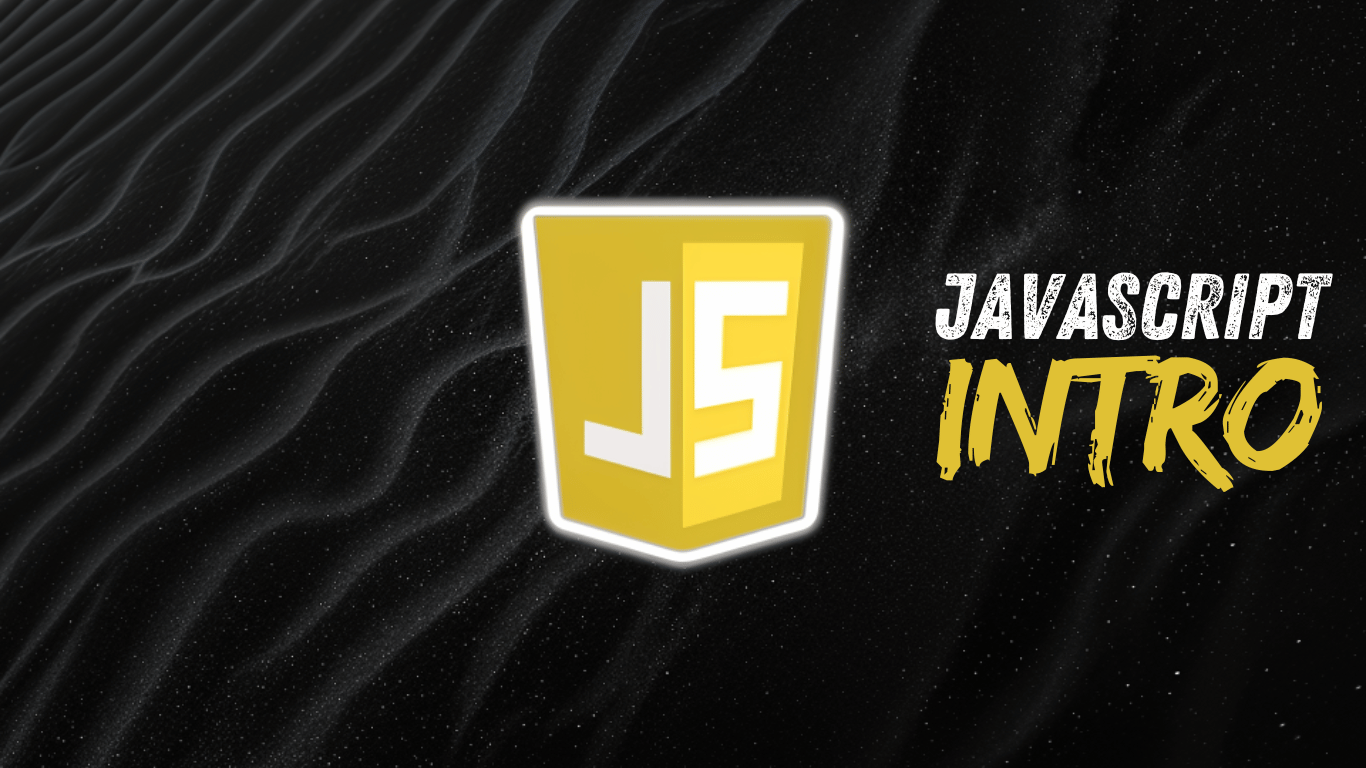
Introduction to JavaScript : the programming language that brings websites to life! Whether you’re fascinated by dynamic web pages or curious about interactive features, JavaScript is the magic that transforms static HTML into an engaging, interactive experience. In this fun-filled guide, we’ll explore why JavaScript is indispensable for modern web development, how it integrates seamlessly with other technologies, and introduce you to its most exciting features. With a splash of humor and short, snappy examples, this overview will get you excited about mastering the language that powers some of the web’s most stunning features.
Table of Contents
Introduction to JavaScript , Why ? The Superhero of Web Development :
Imagine the web as a bustling metropolis. HTML is the city’s sturdy infrastructure, CSS adds the stylish decor, but JavaScript? JavaScript is the superhero that brings everything to life. Here’s why JavaScript is your go-to language:
1. Dynamic Web Content: JavaScript allows you to create interactive elements on your web pages. Think of it as the magic wand that animates your website, making it not just a place to read but a place to experience.
2. User Interaction: Ever clicked a button and seen something happen immediately? That’s JavaScript in action, handling user interactions and responses in real-time.
3. Versatility: JavaScript isn’t just for browsers anymore. With environments like Node.js, you can run JavaScript on servers, too. It’s the Swiss Army knife of programming languages.
4. Rich Ecosystem: The JavaScript ecosystem is enormous. Libraries like React and frameworks like Angular provide powerful tools to streamline your development process.
A Global Overview of JavaScript :
Let’s dive into the enchanting world of JavaScript with a lighthearted script that showcases its core features. We’ll keep it fun, brief, and packed with examples to illustrate how JavaScript works its magic.
The basics : Variables and Data Types :
As an Introduction to JavaScript , variables and containers are essential things to know. These are like the treasure chests where you store your precious information.
Example :
// Meet JavaScript's Characters
// Variables: The Treasure Chests
let heroName = "Captain Code";
let heroPower = 9001; // Power level that exceeds expectations!
console.log(`Our hero is ${heroName} with a power level of ${heroPower}.`);
In this example, heroName and heroPower are variables holding a string and a number, respectively. The console.log function prints them to the screen, showing off our superhero’s stats.
Functions : The magic spells :
Functions are like magic spells that perform tasks when called upon. You define a spell (function) and then cast it (invoke it) whenever needed.
Example :
// The Magic Spell
function castSpell(spellName) {
console.log(`${spellName} has been cast with great power!`);
}
// Casting the Spell
castSpell("Invisibility");
Here, castSpell is a function that takes a spellName and logs a message. When we call castSpell(“Invisibility”), it performs its magic and shows us the result.
Control flow : The decision-makers :
Control flow statements—like if, else, and switch—help your code make decisions, just like a wise sage deciding the fate of your web page.
Example :
// The Wise Sage Decision
let age = 18;
if (age >= 18) {
console.log("You are an adult now! Welcome to the club.");
} else {
console.log("Enjoy your youth while it lasts!");
}
In this snippet, the if statement checks the value of age and prints a message based on whether the age is 18 or older.
Loops : The repetition wizards :
Loops allow you to repeat tasks. They’re like those repetitive spells you cast until you get tired, but in code form!
Example :
// The Repetition Spell
for (let i = 0; i < 5; i++) {
console.log(`Casting spell number ${i + 1}`);
}
The for loop here repeats the spell-casting five times, showing it can handle repetitive tasks efficiently.
Arrays : The Magical Lists :
Arrays are like magical lists where you can keep multiple items. They’re perfect for organizing spells, characters, or any collection of items.
Example :
// The Magical List
let spells = ["Invisibility", "Fireball", "Teleportation"];
console.log(`Our spellbook includes: ${spells.join(", ")}`);
In this example, spells is an array holding different spell names. We use join(“, “) to display them as a comma-separated list.
Objects : The Magical Containers :
Objects are like magical containers that group related data and functions. They’re perfect for keeping track of complex entities.
Example :
// The Magical Container
let wizard = {
name: "Gandalf",
powerLevel: 10000,
castSpell: function() {
console.log(`${this.name} casts a powerful spell!`);
}};
console.log(`${wizard.name} has a power level of ${wizard.powerLevel}.`);
wizard.castSpell();
Here, wizard is an object with properties (name, powerLevel) and a method (castSpell). The this keyword refers to the object itself, allowing the method to access its properties.
The Web Wizardry : DOM Manipulation :
JavaScript can also interact with the HTML of your web page through the Document Object Model (DOM). It’s like casting spells directly on your website!
Example :
// HTML: <button id="magicButton">Click me!</button>
// JavaScript Spell for DOM Manipulation
document.getElementById("magicButton").addEventListener("click", function() {
alert("You’ve unlocked a secret spell!");
});
In this example, when the button with the ID `magicButton` is clicked, an alert pops up. This demonstrates how it can make web pages interactive.
JavaScript is the language that adds magic and interactivity to the web. From variables and functions to loops and objects, it’s the enchanting tool that turns static pages into dynamic experiences. With its ability to interact with the DOM and its rich ecosystem, JavaScript is a must-learn for anyone interested in web development.
Whether you’re new to programming or looking to add a new skill to your arsenal, JavaScript offers endless possibilities for creating engaging and interactive websites. So grab your coding wand and start exploring this wondrous world —your web adventures await!