Mastering JavaScript Basics: Variables and Data Types
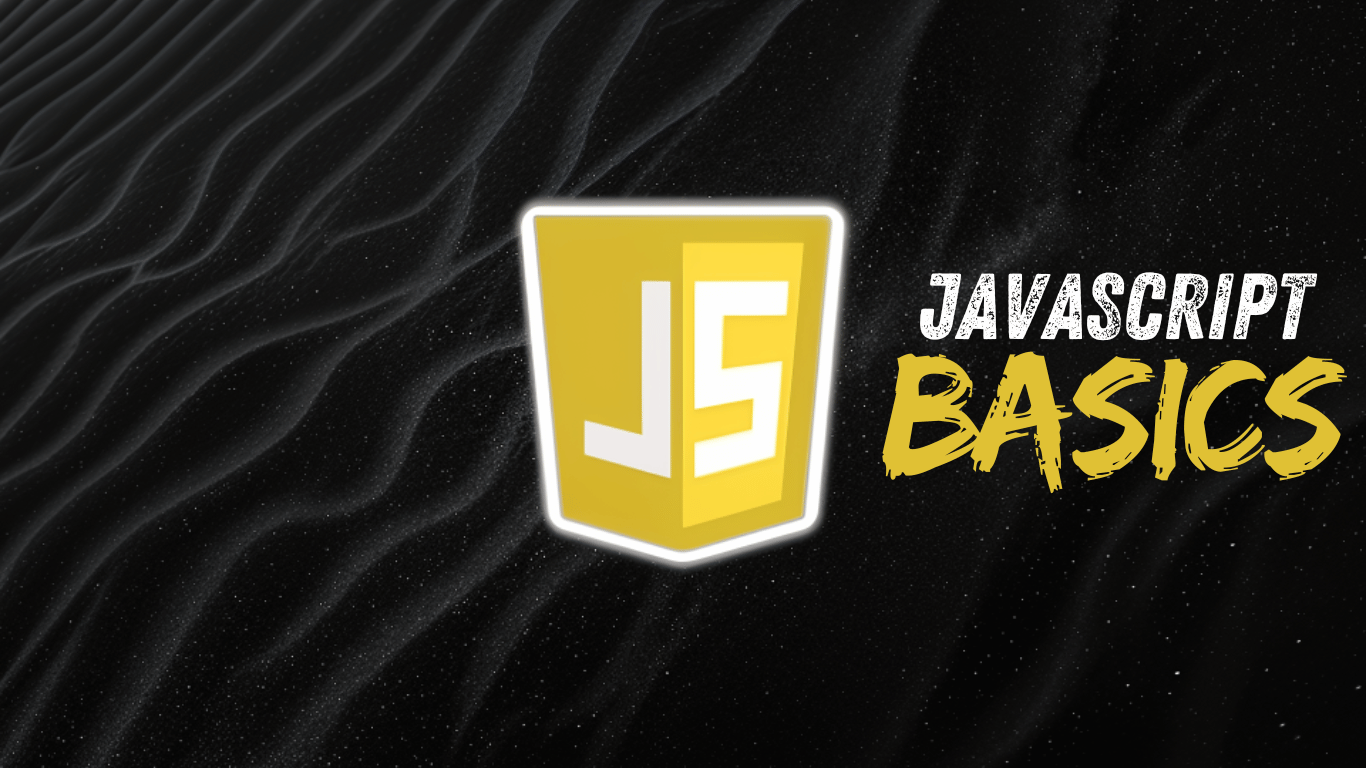
Welcome to the colorful world of JavaScript Basics! If you’ve ever wondered how websites can dance, sing, and react to your clicks, JavaScript is the magic behind the scenes. In this article, we’re diving into the core concepts of JavaScript’s basic syntax and variables. Get ready for an entertaining journey where we’ll turn dry concepts into lively examples, break down the essentials, and sprinkle in some humor to keep things fun and engaging. By the end, you’ll have a strong grasp of JavaScript Basics and how they power dynamic web pages!
Table of Contents
JavaScript Basics : Syntax :
Before we start casting JavaScript spells, let’s get familiar with its syntax—the set of rules that govern how we write code. Think of JavaScript syntax as the grammar of our coding language. Just like in English, if you don’t follow grammar rules, your sentences might not make sense. The same goes for JavaScript!
Example :
// The Basics
let greeting = "Hello, JavaScript!";
console.log(greeting);
In this example, let is used to declare a variable named greeting and assign it the value “Hello , JavaScript!” . The console.log() function then prints this message to the console. It’s like shouting “Hello!” from the code to your browser’s console.
Keywords to Know :
- let : Used to declare variables
- console.log() : Displays information in the console
Declaring Variables in JavaScript : Meet Your New Best Friends :
Variables are like treasure chests where you store your precious data. In JavaScript, you can create these chests using let, const, or var. Think of let and const as modern treasure chests, while var is the old, rusty chest that’s best left in the attic.
1. Using ( let ) : Great for values that might change
2. Using ( const ) : Perfect for values that don’t change , like constants in math .
3. Using ( var ) : An old-school way to declare variables . It’s still around but can be a bit unpredictable .
Example :
// let
let age = 25;
console.log("Age:", age); // Output: Age: 25
// const
const pi = 3.14159;
console.log("Value of Pi:", pi); // Output: Value of Pi: 3.14159
//var
var name = "Alice";
console.log("Name:", name); // Output: Name: Alice
Keywords to Know :
- let : Block-scoped variable .
- const : Block-scoped , read-only variable .
- var : Function-scoped variable .
Data Types : The Building Blocks of Your Code :
JavaScript data types are like the different types of LEGO blocks you use to build awesome creations. Each type serves a specific purpose and has its own quirks. Here’s a quick guide to the most common data types:
- Strings : Sequences of characters, like words or sentences. They’re enclosed in quotes, making them easy to identify.
- Numbers : Represent both integers and floating-point numbers (decimals). They’re used for arithmetic operations.
- Booleans : True or False values . They’re like the binary switch for your logic
- Objects : Collections of key-value pairs. They’re like the ultimate toolbox, where you can store related data.
- Arrays : Lists of values . They’re handy for storing multiple items in a single variable
- Null and Undefined : Special types representing “no value” and “unknown value”. They’re like the mysterious voids in your code.
// Strings
let message = "Welcome to JavaScript!";
console.log(message); // Output: Welcome to JavaScript!
// Numbers
let count = 42;
let price = 19.99;
console.log("Count:", count); // Output: Count: 42
console.log("Price:", price); // Output: Price: 19.99
// Booleans
let isJavaScriptFun = true;
let isPythonBetter = false;
console.log("Is JavaScript fun?", isJavaScriptFun); // Output: Is JavaScript fun? true
// Objects
let car = {
make: "Toyota",
model: "Camry",
year: 2021 };
console.log("Car details:", car); // Output: Car details: {make: "Toyota", model: "Camry", year: 2021}
// Arrays
let colors = ["red", "green", "blue"];
console.log("Colors:", colors); // Output: Colors: ["red", "green", "blue"]
// Null and Indefined
let unknownValue;
let noValue = null;
console.log("Unknown Value:", unknownValue); // Output: Unknown Value: undefined
console.log("No Value:", noValue); // Output: No Value: null
Keywords to know :
- String: Text data.
- Number: Numeric data.
- Boolean: True/false values.
- Object: Key-value pairs.
- Array: List of values.
- Null: Explicitly no value.
- Undefined: Uninitialized value.
Conversion and Template Literals :
JavaScript can convert between different data types automatically, like a chameleon changing colors. This process is known as type coercion.
– Type Coercion: Automatic conversion of data types.
Template literals, introduced in ES6, make string manipulation easier and more readable. They’re like magic scrolls where you can insert variables directly into strings.
– Template Literals: Strings with embedded expressions.
You’re Ready to Code !
Now that you’ve learned the foundational elements of JavaScript, you’re well on your way to becoming a skilled coder. Think of this as the first step in an exciting journey, like picking up a new hobby that opens up endless possibilities. With these key concepts under your belt, you’ll soon be able to dive into more complex and powerful aspects of JavaScript, tackling more challenging projects with confidence.
The fundamental concepts you’ve learned so far serve as your tools, like trusty companions by your side as you explore the language. Once you understand how these building blocks fit together, you’ll be able to write cleaner, more efficient code and approach problems in new, creative ways. You’ll also gain a deeper understanding of how JavaScript can make web pages interactive and dynamic, bringing your ideas to life in unique and engaging ways.
So, with your new knowledge in hand, it’s time to start experimenting and applying what you’ve learned. Try different examples, build small projects, and let your creativity flow! Remember, every expert coder started with the basics, and mastering these will pave the way for success in more advanced topics. The world of JavaScript is full of endless opportunities to learn, grow, and create something exciting. Happy coding, and enjoy the ride as you embark on your JavaScript adventure!