JavaScript: The Basics of Event Handling
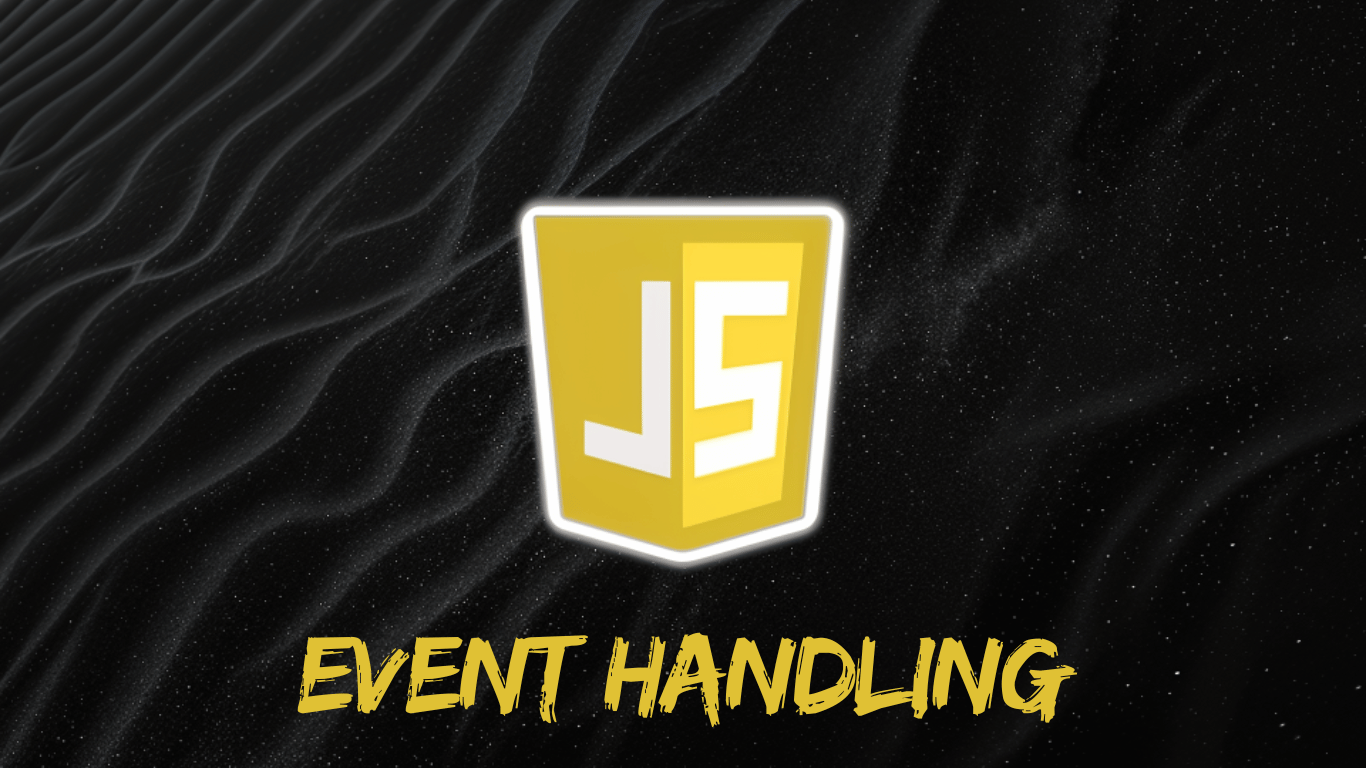
Once upon a time, websites were mere static pages. You could scroll, read, and maybe click on a few links, but they didn’t do much more than that. Now, thanks to the magical powers of JavaScript, websites have become a wonderland of interactivity. This transformation is all thanks to event handlers. These little heroes allow developers to make web pages respond to users in fun, dynamic ways. Every time you click a button, submit a form, or wave your mouse around like a wizard casting a spell, you’re interacting with JavaScript events.
Understanding how events work and how to harness them is essential for any developer who wants to create modern, interactive web applications. So, grab your virtual wand, and let’s dive into the world of JavaScript event handling—where clicks turn into actions and forms come to life !
Table of Contents
What Are Events in JavaScript ?
In the world of JavaScript, an event is simply something that happens on a webpage. It’s like an action that occurs when the user interacts with the page in some way. This could be something as simple as a click, a keypress, or even a page finishing its load. When an event happens, it’s similar to a fire alarm going off, signaling that something has occurred. JavaScript then acts like a superhero, rushing in to handle the situation and make sure everything works smoothly.
To understand this better, let’s imagine you’re at a carnival, and there’s a game where you hit a bell with a hammer. Every time you hit the bell, something happens: lights flash, bells ring, and maybe you even win a stuffed bear! In the world of JavaScript, hitting the bell is the event. Then, after the bell is struck, JavaScript’s event handler takes over and carries out whatever actions are needed, just like the lights flashing or the bell ringing. So, the event (hitting the bell) triggers a response (the lights, bells, or stuffed bear).
JavaScript handles many types of events, and they can be grouped into different categories. Here are some common ones:
- Keyboard and touch events: These happen when you type on a keyboard or tap on a phone screen. It’s like playing a piano—every key press is an event that JavaScript can respond to.
- Click events: This is probably the most familiar type of event. When you click a button or any clickable area on a webpage, something happens. It’s like opening a treasure chest. The chest might not be filled with gold, but instead, JavaScript will trigger whatever action was programmed, like showing a new page or opening a form.
- Form events: Forms are everywhere on websites. When you fill out a form and press the submit button, it’s like handing in your homework. JavaScript then takes over to check the form, process it, and send the data where it needs to go.
- Mouse hover events: Have you ever moved your mouse over an object on a webpage and seen it change color? That’s a mouse-hover event in action. It’s like magic that happens whenever your mouse is over something, and JavaScript responds by making that object change in some way, such as altering its color or shape.
In JavaScript, events are essential because they allow you to interact with the webpage. Whether it’s typing, clicking, submitting a form, or even just hovering your mouse, events are the actions that trigger responses, and JavaScript is always ready to step in and handle them.
Event Listeners : The Eager Eavesdroppers of JavaScript :
Event listeners are a key part of JavaScript, acting like little watchers that stay ready to respond whenever something happens on a webpage. Think of them like a waiter in a restaurant who’s always keeping an eye out for your next request. The event listener just waits, ready for something to happen, like a button click or a mouse move. When an event does occur, the listener jumps into action to make something happen in response.
Sometimes, people mix up event listeners with event handlers, but there’s a simple way to understand the difference. The event listener is like the waiter who’s waiting and watching for an event to happen. The event handler is like the chef in the kitchen, who starts working to prepare a response to the event. They work together like a perfect team: the listener watches, and when an event happens, the handler does the job needed.
To work with event listeners in JavaScript, you typically use two methods : addEventListener and removeEventListener. The addEventListener method is like hiring a waiter to watch for events. When you don’t need the listener anymore, you can call removeEventListener to send them on vacation and stop watching for events. These methods help you control when and how the listener reacts to events on your webpage.
The Event Object : The Sherlock Holmes of Event Handling :
Whenever an event happens on your webpage, JavaScript gathers all the information it needs into something called an event object. Think of it as a detailed report that tells JavaScript exactly what just happened. It’s like a detective notebook that records the who, what, where, and when of the event. Imagine you just clicked a big red button on your screen—JavaScript creates an event object that knows not only which button you clicked but also what type of click it was, and even where your mouse pointer was at that exact moment (though it won’t know if you were smiling or frowning when you clicked, that’s still private!).
This event object is packed with helpful information, making it easier for developers to respond to user interactions and build engaging websites. Here are a few key properties of the event object you’ll want to keep in mind:
- Target: This tells JavaScript exactly which element the event happened on. It’s like saying, “Hey, I clicked that button!” Whether it’s a form, a picture, or a link, the target property has your back.
- Type: What kind of event triggered this? Was it a click, a key press, a double tap, or a swipe? This property spills the beans on the nature of the interaction.
- Keycode: If it’s a keyboard event, this property reveals exactly which key was pressed. Imagine it as finding out the secret password to unlock a door—it helps you react differently based on whether the user pressed Enter, the spacebar, or another key.
By using the event object, developers gain precise control over how their websites respond to different user actions. This makes the web experience smoother, smarter, and way more interactive for users. So, the next time you think about building a web project, remember—the event object is your best friend when it comes to keeping things dynamic and responsive.
Mastering JavaScript Events: Controlling Everything with Ease
JavaScript events are the foundation of making web pages interactive and dynamic. They represent user interactions, such as clicking buttons, submitting forms, or moving the mouse. Mastering how to handle these events effectively can elevate your web development skills, making your websites feel alive and intuitive. Let’s break down some key concepts and learn how to take full control of events with simple and effective techniques.
How to Stop the Browser’s Default Actions
Imagine clicking a link or pressing a button on a webpage, only for the entire page to reload or redirect when that’s not what you wanted. This is the browser’s default behavior, and while it’s helpful in some cases, it can get in the way when you want something custom to happen instead.
For example, when submitting a form, the browser’s default action is to reload the page. But what if you wanted to display a success message without refreshing the page? That’s where JavaScript’s preventDefault() comes to the rescue.
By using preventDefault() , you can stop the browser from performing its usual action. Instead, you take full control and decide what happens next. Here’s an example:
document.querySelector("form").addEventListener("submit", function (event) {
event.preventDefault(); // Stops the page from reloading
alert("Form submitted successfully!");
});
This method ensures you maintain control over what happens during specific interactions. It’s especially useful in modern web applications, where seamless user experience is key.
Think of it like politely telling the browser, “Thanks, but I’ll handle this myself.” Whether you’re building custom forms, buttons, or navigation systems , preventDefault() is a tool you’ll rely on often.
A Smart Way to Handle Multiple Buttons
Imagine you’re building a webpage with a lot of buttons—maybe 50, 100, or even more. Would you attach a separate event listener to every single button? That’s not practical or efficient. Instead, you can use a clever trick called event delegation to manage all the buttons with just one event listener.
Here’s how it works: Instead of adding an event listener to each button, you attach one to a common parent element, such as a container. Then, when any button is clicked, the event “bubbles up” to the parent, where the single event listener can handle it.
document.querySelector(".button-container").addEventListener("click", function (event) {
if (event.target.tagName === "BUTTON") {
alert(`Button clicked: ${event.target.textContent}`);
}
});
In this example, even if there are hundreds of buttons inside the .button-container, you only need one event listener. This makes your code simpler, more efficient, and easier to manage.
Think of it like a carnival where one manager oversees all the games instead of hiring individual attendants for each one. It saves resources and ensures everything runs smoothly.
Event delegation isn’t just a convenience—it’s a best practice for handling events in large, complex web pages.
Tips for Better Event Handling
When working with JavaScript events, keeping your code clean and efficient is just as important as making it functional. Here are some simple yet powerful tips to handle events like a pro:
- Group Related Events: If multiple elements trigger similar actions, try combining them into a single event listener. This keeps your code organized and reduces redundancy.
- Think Before Adding Listeners Everywhere: Avoid attaching event listeners to every element individually, especially if there are many of them. Use techniques like event delegation to save time and improve performance.
- Remove Listeners When You Don’t Need Them: If an element is removed from the page or an event is no longer relevant, clean up the associated event listener to prevent memory leaks and keep your webpage fast.
- Use Meaningful Function Names: Instead of writing anonymous functions everywhere, use descriptive names for event-handling functions to make your code easier to read and debug.
By following these practices, your code will not only work well but will also be easier to maintain, even as your project grows larger and more complex.
By understanding how to manage events effectively—whether it’s stopping default behaviors, handling multiple elements efficiently, or writing clean and maintainable code—you can create interactive and user-friendly web experiences. Once you’ve mastered these techniques, JavaScript events will feel less like a challenge and more like a tool you can bend to your will.
Conclusion :
Event handling in JavaScript is the secret ingredient that turns dull, static websites into vibrant, interactive experiences filled with energy and engagement. Think of it as running your own carnival of digital fun, where clicks, touches, and keystrokes are the attractions that keep users entertained. When a user clicks a button, fills out a form, hovers over an image, or presses a key, these interactions trigger events that can set off custom actions — from displaying playful animations to loading new content seamlessly.
Mastering event handling not only allows you to add dynamic features but also helps create a seamless and intuitive user experience. Whether you’re adding a simple button click handler or crafting intricate custom events that sync animations and effects across a page, knowing how to handle events properly is key. It enables you to control every user interaction and ensures your websites remain fun, functional, and responsive to user actions. In short, understanding JavaScript events empowers you to craft web experiences that are delightful, engaging, and unforgettable.