JavaScript : Introduction to the Document Object Model (DOM)
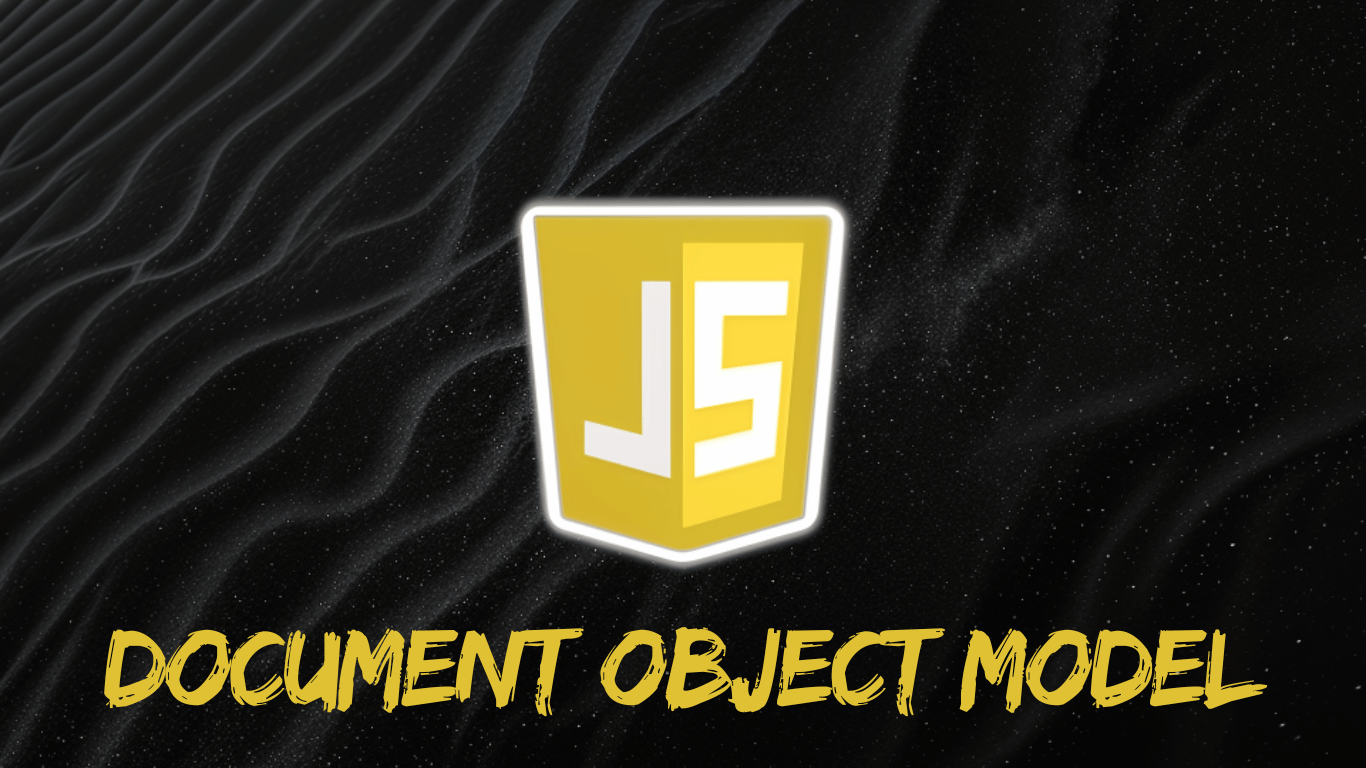
The Document Object Model (DOM) is one of the most important concepts in web development, especially when working with JavaScript. It’s the bridge that allows JavaScript to interact with and manipulate web pages, turning static HTML into dynamic and interactive experiences. If you’ve ever wondered how a button click can trigger a new image to appear or a form to validate itself, the answer lies in the DOM. In this article, we will explore the fundamentals of the DOM, how it works with JavaScript, and the role it plays in creating dynamic web content.
Table of Contents
What is the DOM ?
The Document Object Model (DOM) is a crucial programmatic representation of a web page that enables dynamic interaction and manipulation of web content. When a browser loads an HTML document, it doesn’t just display the static content directly—it creates an internal, structured representation of that document. This representation, commonly referred to as the DOM, is organized as a hierarchical tree of objects, where each object corresponds to specific elements, attributes, or text content from the original HTML document.
The DOM tree structure consists of interconnected nodes that represent various parts of the web document. These nodes fall into different categories, including element nodes, text nodes, attribute nodes, and document nodes. Think of each node as a container or box, which may contain other nested boxes inside it. For instance, an HTML element such as <ul>
(unordered list) may have child nodes like individual <li>
(list item) elements. This parent-child relationship between nodes forms a tree-like structure that allows browsers to render and manage complex webpage layouts.
What makes the DOM so powerful is its dynamic nature. Developers can use programming languages—most commonly JavaScript—to interact with and manipulate the DOM. Through JavaScript, you can add new elements, modify existing ones, change styles, update content, and respond to user actions such as clicks, form submissions, or scrolling. These manipulations make it possible to create modern, interactive web experiences.
For example, imagine a simple interactive feature like a “Read More” button that expands hidden text on a webpage. This functionality is made possible through DOM manipulation. JavaScript can be used to listen for the button click event and then modify the DOM tree to reveal the hidden content by changing its visibility or inserting additional nodes.
The power of the DOM extends beyond just creating interactive features. It plays a critical role in accessibility, search engine optimization (SEO), and rendering performance. Understanding the structure of the DOM and how to efficiently manipulate it is a fundamental skill for web developers.
In summary, the DOM acts as the bridge between web content and user interactivity. It provides a standardized way for programming languages to interact with a webpage’s structure and style. Whether you’re updating the color of a button, validating form inputs, or dynamically loading new content without refreshing the page, you’re leveraging the capabilities of the DOM to deliver a seamless and engaging user experience.
How JavaScript and the DOM Work Together
JavaScript is one of the most popular programming languages for the web. One of the key reasons for its popularity is its ability to interact with web pages in real time, making them dynamic and engaging. But how does this magic happen? That’s where the DOM (Document Object Model) comes into play.
The DOM acts as a bridge between JavaScript and the HTML content of a webpage. Think of the DOM as a blueprint or map of the entire webpage. When a browser loads a website, it creates this structured representation, turning elements like headings, paragraphs, buttons, and images into objects that JavaScript can easily manipulate.
How the DOM Works
Imagine you’ve created a simple HTML page with a couple of paragraphs and a button. Initially, this page is static — nothing changes when a visitor looks at it. The content just sits there.
But when you introduce JavaScript and connect it to the DOM, everything changes. JavaScript can:
- Detect events: For example, it can “listen” for user interactions like mouse clicks, key presses, or form submissions.
- Change content: It can update the text inside paragraphs, headers, or buttons.
- Modify styles: JavaScript can dynamically change colors, fonts, and layout styles to enhance user experience.
- Add or remove elements: Need to show a new message, insert a table, or even hide a section? JavaScript can handle it effortlessly.
Practical Example
Let’s say you want your button to update the text of a paragraph when clicked:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple DOM Interaction</title>
<style>
p {
color: blue;
}
</style>
</head>
<body>
<p id="myParagraph">This is the original text.</p>
<button id="myButton">Click Me!</button>
<script>
// Select the button and paragraph using DOM methods
const button = document.getElementById('myButton');
const paragraph = document.getElementById('myParagraph');
// Add a click event listener to the button
button.addEventListener('click', function() {
paragraph.textContent = 'You just clicked the button!';
paragraph.style.color = 'red'; // Change the text color dynamically
});
</script>
</body>
</html>
In this simple example:
- The DOM allows JavaScript to select elements (
getElementById
). - The button listens for clicks with
addEventListener
. - When clicked, the paragraph text is updated, and its color changes dynamically.
Why is This Important?
Before JavaScript and the DOM, web pages were static and less interactive. With this powerful combination, developers can now create sophisticated web applications like real-time chat systems, dynamic forms, and interactive dashboards.
Learning how to work with the DOM is essential for anyone interested in web development. By mastering it, you’ll unlock the full potential of JavaScript to build engaging, user-friendly websites.
Anatomy of the DOM: Understanding Its Building Blocks
At its core, the DOM represents the structure of an HTML or XML document as a hierarchical tree of nodes. Think of it as a detailed map where every element, piece of text, and attribute becomes a part of this living structure that JavaScript can manipulate.
The key types of nodes you should know are:
- Element Nodes
- Represent the HTML elements in the document, such as
<div>
,<a>
,<ul>
, or<button>
. - These nodes can act as parents and contain child nodes, forming a nested hierarchy.
- For example, a <ul> can contain several <li> elements as its children.
- Represent the HTML elements in the document, such as
- Text Nodes
- Represent the literal text content inside elements, such as the words within a paragraph ( <p> ).
- Every bit of visible text on the page is wrapped in a text node.
- Attribute Nodes
- Represent attributes of HTML elements, such as class , id , href or src .
- JavaScript can read and modify these attributes to change the behavior or appearance of elements dynamically.
Navigating and Manipulating the DOM with JavaScript
At the heart of working with the DOM is the document object, which represents the entire web page. Once you have access to the document , you can explore and manipulate its nodes in a variety of ways.
Here are some common tasks and how they’re accomplished:
1. Selecting Elements
You can use methods like getElementById(), querySelector(), and querySelectorAll() to select elements.
Example: Selecting a paragraph and changing its text:
const paragraph = document.getElementById('myParagraph');
paragraph.textContent = 'This text was changed by JavaScript!';
2. Modifying Styles
Want to change how an element looks? JavaScript can modify the CSS styles of any element.
const button = document.querySelector('button');
button.style.backgroundColor = 'green';
3. Listening for Events
You can make elements respond to user actions like clicks, key presses, or mouse movements.
Example: Changing text color when a button is clicked:
button.addEventListener('click', function() {
paragraph.style.color = 'blue';
});
4. Adding or Removing Elements Dynamically
JavaScript can create new elements and insert them into the DOM or remove existing ones.
const newElement = document.createElement('p');
newElement.textContent = 'Hello, this is a new paragraph!';
document.body.appendChild(newElement); // Adds the new paragraph to the page
Bringing Pages to Life: Real-world Example
Imagine you have a button on your page labeled “Show Secret Message.” You want this button to reveal a hidden message when clicked. Here’s a simple way to do it using JavaScript and the DOM:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Secret Message Example</title>
<style>
#secret {
display: none;
color: red;
font-weight: bold;
}
</style>
</head>
<body>
<button id="revealButton">Show Secret Message</button>
<p id="secret">This is the secret message!</p>
<script>
const button = document.getElementById('revealButton');
const secretMessage = document.getElementById('secret');
button.addEventListener('click', function() {
secretMessage.style.display = 'block'; // Reveal the hidden message
});
</script>
</body>
</html>
DOM Elements as Objects and the Role of APIs in Web Development
The Document Object Model (DOM) is a powerful interface that transforms static HTML into dynamic, interactive web experiences. It allows developers to treat elements on a webpage as objects, complete with their own properties and methods. This makes it possible to modify, style, and control elements directly from JavaScript. Additionally, the DOM’s capabilities are extended by various APIs (Application Programming Interfaces), which provide specialized tools for working with different types of content, such as HTML structures, CSS rules, and SVG graphics.
DOM Elements as Objects
One of the most powerful aspects of the DOM is its object-oriented nature. Every element in the document is treated as an object, meaning it comes with properties that store information and methods that perform actions.
Key Properties
- innerHTML: Stores the HTML content inside an element and can be modified to update that content.
- textContent: Contains only the text within an element, excluding any HTML tags.
- style: Provides access to an element’s inline styles, allowing direct modification of visual properties like color and font.
Common Methods
- getAttribute() and setAttribute(): Allow retrieval and modification of element attributes such as IDs and classes.
- appendChild() and removeChild(): Enable dynamic addition or removal of elements from the page structure.
By leveraging these properties and methods, developers can build highly interactive and visually dynamic web pages.
APIs and the DOM
The DOM’s capabilities are built upon a collection of APIs that provide developers with advanced functionality to manipulate and enhance web content. These APIs are essential for creating modern, responsive web applications.
Core DOM API
The fundamental layer that enables developers to traverse the document structure, access elements, and manipulate properties and content.
HTML DOM API
An extension of the core DOM, this API includes tools specifically designed for handling HTML elements, enabling easy manipulation of form inputs, lists, and buttons.
CSSOM (CSS Object Model)
This API provides programmatic access to CSS rules and allows developers to read, modify, or apply new styles dynamically.
SVG DOM API
Designed for working with scalable vector graphics, this API allows the manipulation of graphical properties and shapes in SVG content.
The Power of Combining DOM Objects and APIs
The combination of object-oriented DOM elements and specialized APIs provides developers with an incredibly versatile toolkit for creating dynamic user experiences. By mastering these tools, developers can unlock countless possibilities for enhancing the structure, behavior, and design of their websites.
Conclusion :
The DOM is the backbone of web development, enabling JavaScript to transform static web pages into dynamic, interactive experiences. By understanding how the DOM works and how JavaScript can manipulate it, you gain the ability to create websites that are not only functional but also engaging and responsive. Whether you’re building a simple interactive form or a complex web application, mastering the DOM opens the door to endless possibilities in JavaScript development.