Mastering Arithmetic Operators in C : Full Guide For Beginners
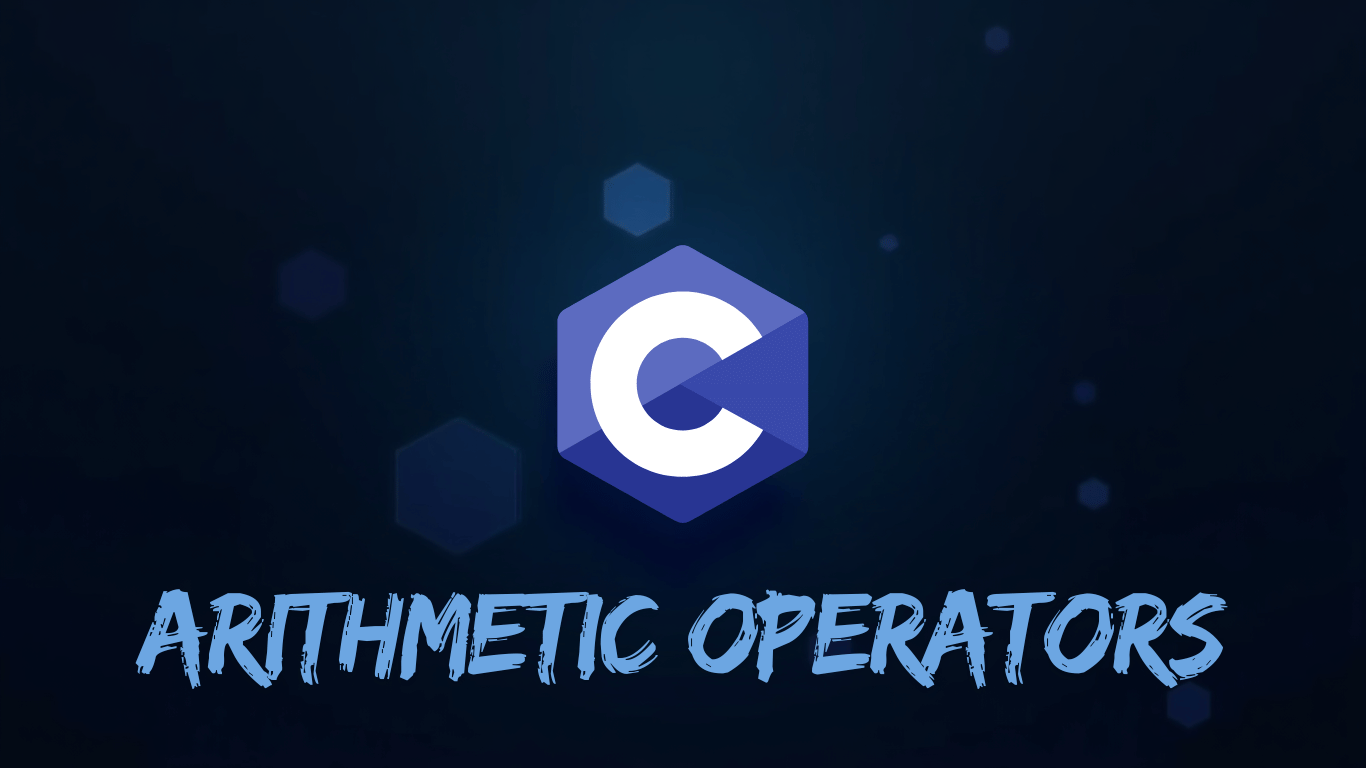
Programming can often feel like a foreign language, especially when you dive into the details of a specific language like C. One of the key elements that makes C both powerful and flexible are its arithmetic operators. These operators are not just simple symbols; they are your gateway to performing mathematical calculations, manipulating data, and bringing your programming ideas to life. This article will guide you through understanding arithmetic operators in C language, equipping you with the knowledge and skills you need to use them effectively in your coding projects.
Table of Contents
What Are Arithmetic Operators in C?
Arithmetic operators are fundamental building blocks in C programming. They allow you to perform various mathematical operations, making them crucial for everything from simple calculations to complex algorithms. In C, these operators handle numerical values (operands) to carry out calculations such as addition, subtraction, multiplication, division, and modulus.
What Is Arithmetic Operator in C?
In the context of C programming, an arithmetic operator is a symbol that instructs the compiler to perform a specific mathematical operation. Understanding these operators is essential for any aspiring programmer, as they form the basis for more complex operations. Whether you’re creating a simple calculator or developing a more intricate software application, arithmetic operators will be at the core of your calculations.
Types of Arithmetic Operators in C Language
C language provides five primary arithmetic operators, each serving a distinct purpose. Let’s take a closer look at these operators and their functionalities.
1. Addition Operator (+)
The addition operator is one of the most commonly used arithmetic operators. It allows you to add two or more operands together.
Usage:
int a = 5;
int b = 10;
int sum = a + b; // sum now holds the value 15
2. Subtraction Operator (-)
The subtraction operator lets you subtract one operand from another. It’s straightforward and essential for calculations that require finding the difference.
Usage:
int a = 15;
int b = 5;
int difference = a - b; // difference now holds the value 10
3. Multiplication Operator (*)
This operator is used to multiply two operands. It’s a crucial operator when working with calculations that require scaling or combining values.
Usage:
int a = 4;
int b = 3;
int product = a * b; // product now holds the value 12
4. Division Operator (/)
The division operator divides one operand by another. It’s important to note that if you divide two integers in C, the result will also be an integer, with any remainder discarded.
Usage:
int a = 20;
int b = 4;
int quotient = a / b; // quotient now holds the value 5
5. Modulus Operator (%)
The modulus operator is used to find the remainder of a division operation. This operator is particularly useful in scenarios where you need to determine if a number is even or odd, or to cycle through values.
Usage:
int a = 10;
int b = 3;
int remainder = a % b; // remainder now holds the value 1
How to Use Arithmetic Operators in C
Using arithmetic operators in C is straightforward once you understand the syntax. You can create expressions involving these operators and assign the results to variables.
Basic Syntax and Implementation
Here’s a simple example that combines several arithmetic operations:
#include <stdio.h>
int main() {
int a = 10;
int b = 5;
int sum = a + b;
int difference = a - b;
int product = a * b;
int quotient = a / b;
int remainder = a % b;
printf("Sum: %d\n", sum);
printf("Difference: %d\n", difference);
printf("Product: %d\n", product);
printf("Quotient: %d\n", quotient);
printf("Remainder: %d\n", remainder);
return 0;
}
Output of the Code
When you run the code above, you’ll see the following output:
Sum: 15
Difference: 5
Product: 50
Quotient: 2
Remainder: 0
Operator Precedence and Associativity
Understanding operator precedence and associativity is crucial for writing accurate and efficient code. Operator precedence determines the order in which operators are evaluated in an expression, while associativity defines the direction in which operators of the same precedence are processed.
Understanding Operator Hierarchy
In C, arithmetic operators have specific precedence levels. Here’s a brief overview of how they rank:
- Multiplication (*), Division (/), Modulus (%) – highest precedence
- Addition (+), Subtraction (-) – lower precedence
Table of Precedence
Operator | Description | Precedence |
---|---|---|
* / % | Multiplication/Division/Modulus | 1 |
+ – | Addition/Subtraction | 2 |
Examples of Precedence
Consider the following expression:
int result = 5 + 2 * 3; // result is 11, not 21
In this case, the multiplication is performed first due to its higher precedence.
Common Errors with Arithmetic Operators
While arithmetic operators are relatively straightforward, beginners often make mistakes that can lead to unexpected results. Here are some common errors to watch out for:
- Integer Division: Remember that dividing two integers results in an integer. For example,
5 / 2
will yield2
, not2.5
. - Using Wrong Operator: Ensure you’re using the correct operator for the operation you intend. Mixing up
*
and+
can lead to significant logical errors. - Remainder Confusion: Be careful with the modulus operator. If you use it with a divisor of zero, it will lead to a runtime error.
Practical Examples of Arithmetic Operators in C
Understanding how to implement these operators in real-world scenarios can significantly enhance your coding skills. Here’s a practical example where arithmetic operators come into play.
Real-World Application: Simple Calculator
You can create a simple calculator program that performs basic arithmetic operations based on user input. Here’s a basic structure for such a program:
#include <stdio.h>
int main() {
int a, b;
char operator;
printf("Enter first number: ");
scanf("%d", &a);
printf("Enter second number: ");
scanf("%d", &b);
printf("Enter operator (+, -, *, /, %%): ");
scanf(" %c", &operator);
switch (operator) {
case '+':
printf("Result: %d\n", a + b);
break;
case '-':
printf("Result: %d\n", a - b);
break;
case '*':
printf("Result: %d\n", a * b);
break;
case '/':
if (b != 0) {
printf("Result: %d\n", a / b);
} else {
printf("Error: Division by zero\n");
}
break;
case '%':
if (b != 0) {
printf("Result: %d\n", a % b);
} else {
printf("Error: Division by zero\n");
}
break;
default:
printf("Invalid operator\n");
break;
}
return 0;
}
Code Explanation
This calculator program prompts the user to enter two numbers and choose an arithmetic operation. It then performs the operation using a switch
statement and displays the result. This example illustrates how arithmetic operators can be applied interactively, making it a practical demonstration of their functionality.
Conclusion
Mastering arithmetic operators in C is an essential skill that opens the door to more complex programming concepts. By understanding how to use these operators effectively, you can enhance your coding projects and tackle various mathematical challenges with confidence. Whether you’re developing a simple calculator or diving into more advanced programming tasks, arithmetic operators will play a crucial role in your success.
Call to Action
Now that you have a solid understanding of arithmetic operators in C, it’s time to put your knowledge into practice! Start experimenting with your own code snippets, create small projects, or even build a simple calculator. The more you practice, the more proficient you will become. If you have any questions or want to share your coding experiences, feel free to leave a comment below!
Frequently Asked Questions (FAQs)
What is an arithmetic operator in C?
An arithmetic operator in C is a symbol that allows you to perform mathematical operations on operands, such as addition, subtraction, multiplication, division, and modulus.
What are the different types of arithmetic operators in C language?
The primary arithmetic operators in C are addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
How do I avoid errors when using arithmetic operators?
To avoid errors, ensure you understand operator precedence, be careful with integer division, and always check for division by zero when using the division and modulus operators.
By understanding and mastering these concepts, you will greatly enhance your programming capabilities in C, allowing you to tackle more complex problems with ease. Happy coding!