Understanding Data Types in C: A Comprehensive Guide
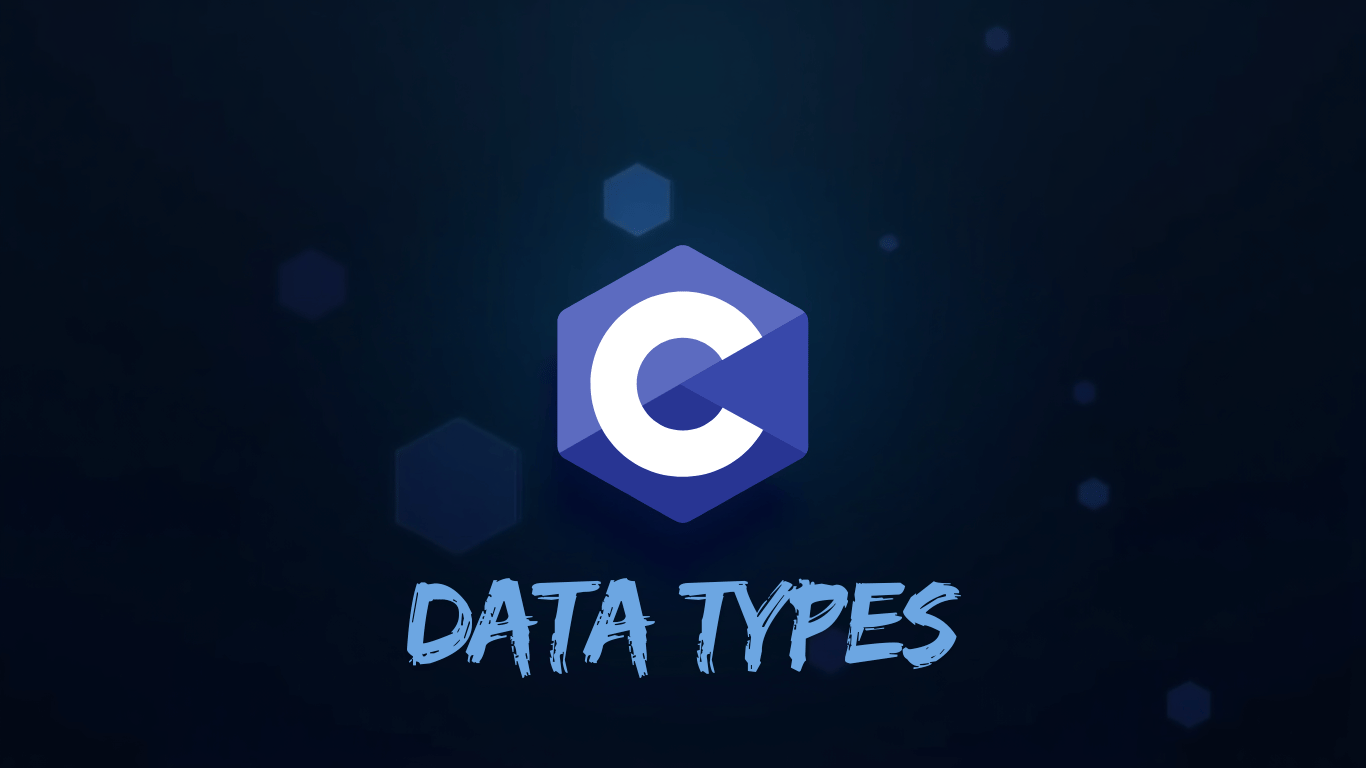
Introduction: Why Data Types Matter in C Programming
When you begin coding in C, one of the first things you encounter is the concept of data types. Imagine trying to store numbers, characters, or decimal values without defining their nature—chaos would ensue. Data types bring order by specifying how much memory a variable occupies and what kind of values it can hold.
Understanding data types in C is essential for efficient memory management and precise calculations. Without them, your program would struggle with errors and unexpected behavior. Whether you’re a beginner or an experienced programmer, mastering data types will set a solid foundation for writing optimized C programs.
Table of Contents
What is Data Type in C?
A data type in C is a classification that determines the type of data a variable can store. It also dictates the amount of memory allocated for that variable and the operations that can be performed on it.
For example, consider this simple declaration:
int age = 25;
Here, int
specifies that the variable age
can only store integer values. Choosing the right data type is crucial for ensuring efficiency and preventing unnecessary memory usage.
Categories of Data Types in C
Primary Data Types in C
C offers several built-in data types, commonly referred to as primary data types. These are the backbone of all variables and functions in C.
Data Type | Description | Example | Memory Size (Approx.) |
---|---|---|---|
int | Stores integer values | int num = 10; | 4 bytes |
float | Stores decimal numbers | float pi = 3.14; | 4 bytes |
double | Stores high-precision floating-point numbers | double g = 9.81; | 8 bytes |
char | Stores single characters | char grade = 'A'; | 1 byte |
void | Represents absence of value | void function(); | – |
Each of these types serves a specific purpose. For instance, float
and double
are used for decimal calculations, while char
holds single characters.
Derived Data Types in C
Derived data types are built upon primary types. They allow for more complex data structures in C programming.
- Arrays – Used to store multiple values of the same type.
int arr[5] = {1, 2, 3, 4, 5};
- Pointers – Variables that store memory addresses.
int num = 10; int *ptr = # // Pointer stores the address of num
- Structures – Custom-defined data types that group related variables.
struct Student { char name[20]; int age; };
- Unions – Similar to structures but with shared memory for all members.
union Data { int i; float f; };
These types enhance flexibility in programming, allowing you to manage data more effectively.
User-Defined Data Types in C
C also allows you to define your own data types using typedef
and enum
.
- Typedef: Creates an alias for an existing type.
typedef unsigned int uint; uint num = 100; // 'uint' is now another name for unsigned int
- Enumerations (
enum
) – Used to define a set of named integer constants.enum Color { RED, GREEN, BLUE };
These help in improving code readability and maintainability.
What is the Difference Between float and double?
float
and double
are both used for storing decimal numbers, but they differ in precision and memory usage.
Feature | float | double |
---|---|---|
Precision | 6-7 digits | 15-16 digits |
Memory | 4 bytes | 8 bytes |
Use Case | When memory is limited | When high precision is needed |
If you need more precise calculations, double
is the better choice.
How to Choose the Right Data Type in C?
Selecting the correct data type depends on several factors:
- Memory efficiency: Use
int
instead ofdouble
when decimal precision isn’t necessary. - Performance: Smaller data types execute faster.
- Accuracy: Choose
double
for scientific calculations. - Portability: Some data types may vary in size across different systems.
For best performance, always consider the trade-off between memory and precision.
Common Errors When Using Data Types in C
Mistakes in selecting and using data types can lead to errors. Here are some common ones:
- Type mismatch: Assigning a
float
value to anint
variable without explicit conversion.int num = 3.14; // Incorrect int num = (int)3.14; // Correct
- Overflow and underflow: Using values that exceed the range of the data type.
- Incorrect type usage in conditions:
if (5.0) { // Condition evaluates to true, but may be unintended printf("This is always true"); }
By understanding these errors, you can avoid bugs in your code.
FAQ – Frequently Asked Questions About Data Types in C
Q1: What is data type in C?
A data type defines the kind of data a variable can store and its memory allocation.
Q2: What are the main data types in C programming?
The primary data types in C include int
, float
, double
, char
, and void
.
Q3: How do I define a data type in C?
You specify the data type when declaring a variable:
int number = 5;
Q4: What is the difference between int and float?
int
stores whole numbers, whilefloat
stores decimal numbers.- Example:
int num = 10; float pi = 3.14;
Q5: Why is void used in C?
void
is used when a function does not return any value.
void greet() { printf("Hello!"); }
Conclusion: Mastering Data Types for Better C Programming
Data types are the foundation of efficient and error-free C programming. Understanding their characteristics enables you to write optimized, scalable, and high-performance programs.
Now that you have a solid grasp of C data types, why not test your knowledge? Try writing a small program using different types and observe how they interact. Happy coding!