JavaScript : Creating Objects
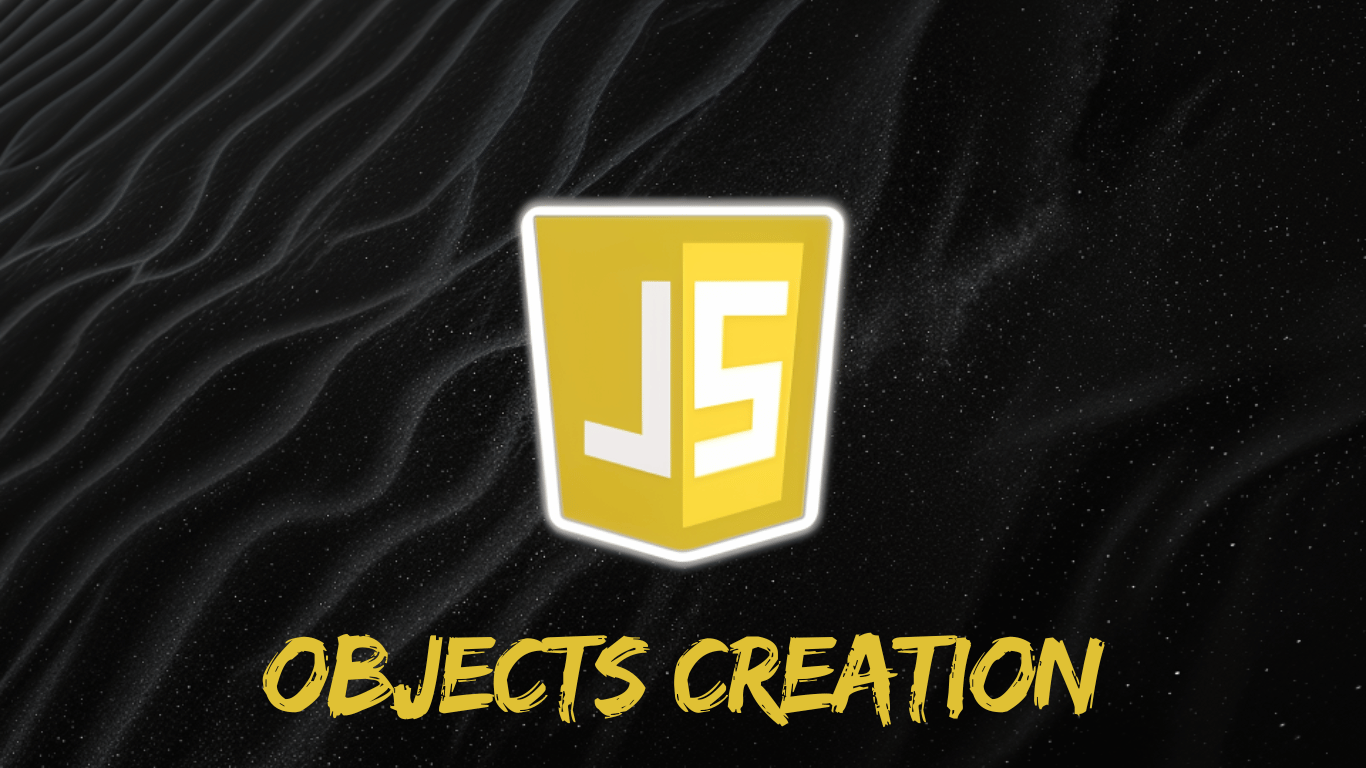
By the end of this guide, you will be equipped to make informed decisions about the most suitable way to create objects in your JavaScript applications. You’ll also learn best practices to ensure your object-oriented code is efficient and well-structured.We start by defining what objects are in JavaScript and discussing their advantages. We then examine different methods for creating objects, such as object literals, constructor functions, and the Object.create() method. Each method is explained in detail to help you understand its usage and purpose.
This article explores the various methods for creating objects in JavaScript, providing you with the knowledge to select the most effective approach for your projects.
In programming, objects are essential for modeling real-world entities or concepts. JavaScript, a versatile and widely-used language, offers multiple ways to create objects, each suited to different needs.
Table of Contents
Understanding Objects in JavaScript
Objects in JavaScript are one of the most fundamental and versatile data structures, designed to store collections of related data and functionality. They provide a way to group multiple pieces of information into a single entity, making it easier to organize and manage complex code.
An object in JavaScript consists of key-value pairs, where the keys are strings or symbols that serve as unique identifiers, and the values can be of any data type. These values can include primitive types like numbers, strings, and booleans, as well as more complex types such as arrays, other objects, and functions (which are often referred to as methods when defined within an object).
Objects are often used to represent real-world entities or abstract concepts in code. For example, you could create an object to represent a “car,” which might have properties like make , model , and
color , along with methods like start() or drive() .
const car = {
make: "Toyota",
model: "Corolla",
color: "Red",
start: function () {
console.log("The car is starting");
},
drive: function () {
console.log("The car is driving");
}
};
car.start(); // Outputs: "The car is starting"
car.drive(); // Outputs: "The car is driving"
In addition to being simple containers for key-value pairs, objects in JavaScript also play a crucial role in advanced programming concepts like prototypal inheritance. This allows objects to share properties and methods through prototype chains, providing a way to extend functionality efficiently without duplicating code.
Thanks to their flexibility and power, objects are widely used throughout JavaScript applications, from representing structured data to building complex components in modern frameworks and libraries like React and Node.js.
Methods for Creating Objects in JavaScript
Using Object Literals in JavaScript
Object literals are one of the simplest and most commonly used ways to create objects in JavaScript. They provide a straightforward syntax that allows developers to define objects directly without needing to use constructor functions or classes. This approach is particularly useful for small, fixed data structures, configuration settings, or when quickly organizing related properties and behaviors.
The syntax for an object literal is defined by curly braces {}
containing one or more key-value pairs, where keys are property names (strings or symbols) and values can be any data type. These values might include numbers, strings, booleans, arrays, other objects, or functions.
const person = {
name: "John Doe",
age: 30,
occupation: "Engineer",
greet: function () {
console.log(`Hello, my name is ${this.name}.`);
}
};
person.greet(); // Outputs: "Hello, my name is John Doe."
Adding Properties and Methods to Object Literals
When defining an object literal, properties and methods can be included directly within the curly braces as key-value pairs. The properties store data, while the methods represent behaviors associated with the object.
Properties:
A property in an object literal is defined by pairing a key with a value. The key serves as the identifier for accessing the value, and the value can be any data type.
Methods:
Methods are functions defined as part of an object. They provide behavior or actions that the object can perform, often utilizing the this
keyword to refer to the object itself.
const book = {
title: "JavaScript for Beginners",
author: "Jane Smith",
read: false,
toggleReadStatus: function () {
this.read = !this.read;
console.log(`Read status: ${this.read ? "Read" : "Unread"}`);
}
};
book.toggleReadStatus(); // Outputs: "Read status: Read"
Nesting Objects and Arrays within Object Literals
JavaScript allows for the creation of complex data structures by nesting objects and arrays within object literals. This approach is particularly useful when modeling hierarchical data or representing multiple entities within a single structure. Nesting enables developers to group related information logically, making the data more organized and easier to access.
When objects are nested, each object can contain its own set of properties and methods, effectively creating sub-objects within the main object. This structure is often used to represent scenarios where a single entity encompasses several smaller components, such as user profiles containing contact information or configuration settings grouped into categories.
Similarly, arrays can be nested within objects to store collections of data related to a particular key. This is useful for representing lists, collections, or sequences of items that belong to a specific entity, such as books in a library, products in a catalog, or tasks in a project management application.
By combining nested objects and arrays, developers can efficiently manage complex datasets, access specific pieces of information, and perform operations on grouped data. This flexibility makes nested data structures an essential tool for creating scalable and well-structured JavaScript applications. Proper design and thoughtful nesting help ensure code maintainability and readability, allowing developers to handle sophisticated data management tasks with ease.
Benefits of Creating Objects in JavaScript
Creating objects in JavaScript offers numerous benefits that contribute to cleaner, more maintainable, and efficient code development. Understanding these advantages can help developers design better applications and data models.
- Organization: Objects provide a structured way to group related data and functions within a single entity. By encapsulating multiple properties and methods, objects allow developers to logically organize code, making it more readable and easier to navigate. This organization is especially beneficial in complex applications where different components must interact seamlessly.
- Encapsulation: One of the key principles of object-oriented programming is encapsulation, which is naturally supported by JavaScript objects. Encapsulation enables developers to bundle both data and behavior together, limiting direct access to the internal state of the object and reducing the risk of accidental interference. This approach promotes modular and maintainable code structures by allowing each object to manage its state independently.
- Reusability: Objects in JavaScript encourage code reusability. Developers can create general-purpose objects that can be reused across different parts of an application, reducing redundancy and improving development efficiency. When combined with inheritance or prototype-based structures, objects become even more powerful in sharing common properties and methods.
- Flexibility: One of the standout features of JavaScript objects is their dynamic nature. Developers can easily add, modify, or delete properties and methods at runtime, making it possible to adapt applications to evolving requirements without significant refactoring. This flexibility is particularly useful when working in rapidly changing environments or when handling user-generated content.
- Efficient Memory Usage and Passing by Reference: Objects in JavaScript are passed by reference, meaning that multiple references can point to the same object in memory. This allows for efficient manipulation of large or complex data structures without unnecessary memory overhead. By referencing the same object, changes made in one part of the code can be reflected elsewhere, reducing the need for extensive data copying.
- Extensibility and Scalability: Objects in JavaScript provide a scalable foundation for building more complex systems. They can be extended by adding new properties and methods as applications grow, enabling developers to build sophisticated and feature-rich solutions without a complete architectural overhaul.
By leveraging these advantages, developers can create robust, scalable, and maintainable applications. The ability to encapsulate data, promote code reuse, and adapt dynamically makes objects a powerful tool in JavaScript development.
Best Practices for Creating Objects in JavaScript
When developing applications with JavaScript, following best practices for creating and managing objects ensures maintainable, scalable, and efficient code. Below are detailed guidelines to help make the most out of JavaScript objects:
- Use Object Literals for Simple, Static Structures:
Object literals provide a straightforward and efficient way to create small, fixed objects that do not require extensive behavior or inheritance. They are ideal for configuration settings, simple data structures, or when organizing related properties in a single container. - Choose Constructor Functions or ES6 Classes for Shared Properties and Methods:
When creating multiple objects that share common characteristics or behaviors, consider using constructor functions or ES6 classes. Constructor functions have been a staple of JavaScript for a long time and remain compatible with older codebases. They enable the creation of reusable object templates that can be instantiated with unique values. - Favor ES6 Classes for Modern JavaScript Development:
ES6 classes offer a cleaner, more intuitive syntax for defining object blueprints, making code easier to read and maintain. They provide familiar object-oriented programming features such as inheritance through the extends keyword and a more concise way to define methods using the class body directly. - Use Factory Functions for Greater Flexibility:
Factory functions are an excellent way to encapsulate object creation logic while returning customized instances. Unlike classes, factory functions do not require the new keyword and offer more flexibility when dealing with conditional properties or complex initialization logic. - Opt for Object.create() for Explicit Prototype Control:
When precise control over an object’s prototype is necessary or when leveraging prototype-based inheritance, Object.create() can be a valuable tool. It allows developers to create objects directly from a specified prototype without relying on constructors or classes. - Encapsulate Initialization Logic:
Whether using constructor functions, classes, or factory functions, encapsulate initialization logic within these structures to keep code modular and maintainable. This ensures that new instances are created with consistent initial states and avoids scattered setup logic throughout the codebase. - Minimize Direct Mutation of Object Properties:
Avoid directly modifying object properties, especially when dealing with shared or global objects, as this can lead to unexpected behaviors and difficult-to-trace bugs. Instead, consider using immutability patterns or employing getters and setters to control access and updates to object properties. - Adhere to Naming Conventions:
Follow established naming conventions for clarity and consistency:- Use PascalCase (capitalized camel case) for constructor functions and classes, such as Person or Animal .Use camelCase (lowercase initial) for factory functions, like createPerson or buildComponent .
- Prefer Composition Over Inheritance:
Composition, where objects are built by combining smaller, specialized components, offers greater flexibility and code reuse compared to traditional inheritance. By focusing on what an object does rather than what it is, composition avoids the pitfalls of rigid inheritance hierarchies. - Document Object Structures, Properties, and Methods:
Proper documentation is crucial for maintaining code and ensuring that other developers (or even your future self) can quickly understand the purpose and functionality of objects. Include clear descriptions of properties, expected types, methods, and usage examples.
By adhering to these best practices, developers can create robust, maintainable, and scalable JavaScript applications that leverage the power of objects effectively. Proper planning, encapsulation, and a consistent approach to object management will lead to cleaner and more efficient code.
Conclusion
This guide has covered various methods for creating objects in JavaScript: object literals, constructor functions, the Object.create() method, and ES6 classes. Each approach has its advantages and use cases.Object literals are great for simple, one-off objects. Constructor functions and classes provide reusable blueprints for objects with shared properties and methods. The Object.create()method offers more control over prototypes and inheritance. By considering best practices, you can leverage JavaScript’s object capabilities to write cleaner, more efficient, and more maintainable code. Choose the approach that best fits your needs, prioritize readability, and refer back to this guide as needed.