Python Comments: The Unsung Heroes of Code
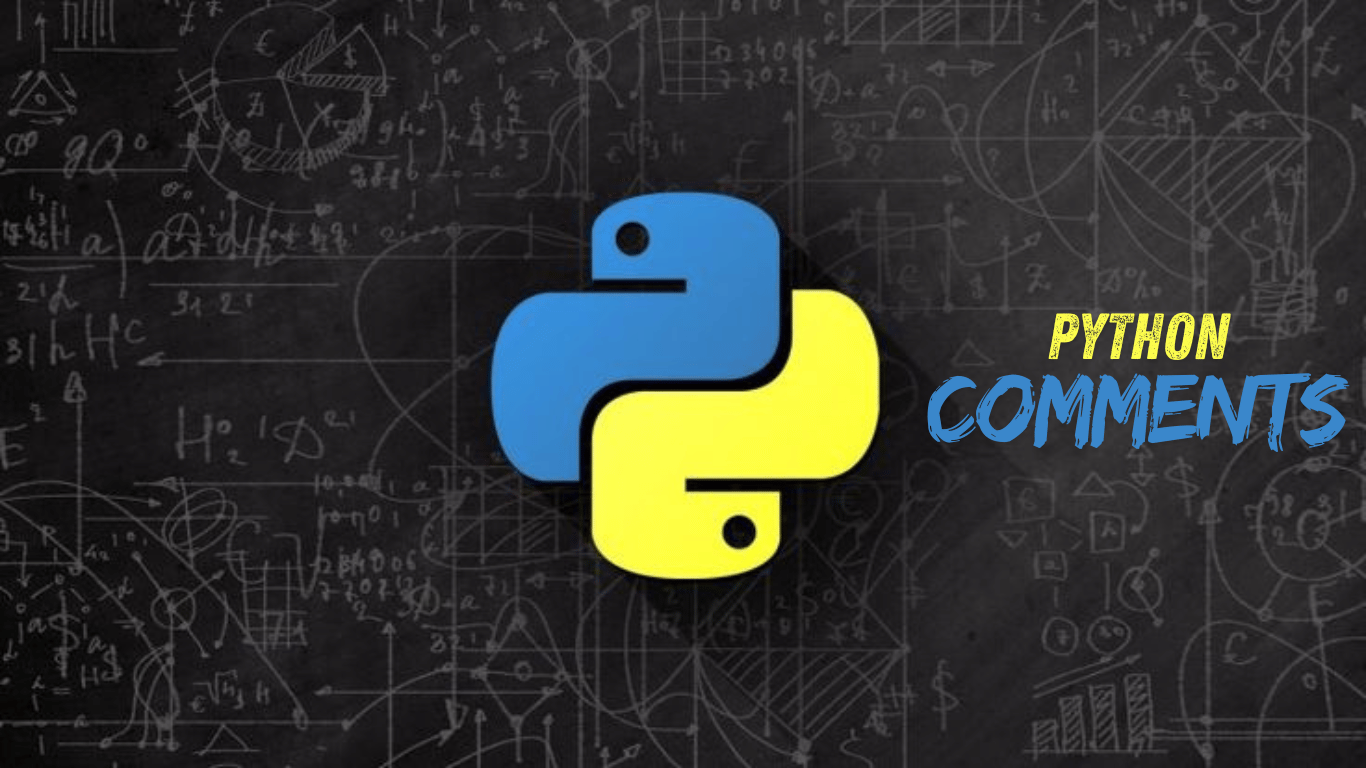
Welcome to the fun and helpful world of Python comments! If you’ve ever seen those little #
symbols or triple quotes in Python and weren’t sure what they do, you’re in the right place. Comments are an important part of coding that make your programs much easier to understand. They don’t affect how your code works or change what your program does—they’re there to explain things.
Whether you’re writing simple reminders to yourself, giving a detailed explanation of a tricky part of your code, or even adding some fun jokes, comments are a powerful tool for making your code better. You might think of them as little notes that help others (or even your future self) understand what’s going on in your program.
In this guide, we’ll dive into how comments work in Python and why they’re so essential for programmers. You’ll learn how to use them effectively, why they make your code clearer, and how they help everyone working with your code—whether you’re working on your own or with a team. Comments are more than just a nice addition to your code; they’re a must-have that can save you a lot of time and frustration in the long run. Let’s get started and explore the world of Python comments!
Table of Contents
What are Python comments ?
In the vast world of programming, comments are like the unsung heroes that work behind the scenes. They’re the friendly notes that programmers leave for themselves, their teammates, or anyone who may be reading or working with their code in the future. These comments don’t change how the code actually runs, so they don’t affect the functionality or performance of the program. Instead, they act as helpful guides, providing explanations, clarifications, and additional context.
Imagine putting together a play or a performance—while the actors on stage are the ones who are seen, there’s a whole backstage crew that makes sure everything runs smoothly. Comments in programming work in much the same way. While your code is the star, the comments are the behind-the-scenes workers who ensure everything is clear and easy to follow. They can explain why certain decisions were made, describe the function of different sections of code, or even offer reminders for things that still need to be done.
But comments aren’t just practical—they can also add a touch of humor or personality to your code. Just like how backstage workers might leave fun notes or create inside jokes, comments can be a place for programmers to share a bit of their creativity or lighten the mood, especially when working on long or complex projects. Whether it’s a funny quip about debugging or a witty remark about a tricky piece of code, comments can make the coding process feel a bit more enjoyable.
At their core, comments are there to make the code more understandable and maintainable. They help others (or your future self) understand why and how something was done, which can save a lot of time and confusion later on. So, while they may not be visible when the code is running, comments are essential for making sure the code is accessible, readable, and a bit more fun.
In Python, comments come in two flavors:
1. Single-Line Comments :
– These start with the # symbol. Everything after the # on that line is a comment.
#This is a single-line comment
print("Hello,World!") #This comment is like a post-it note on the code
2 . Multi-line comments :
– While Python doesn’t have a specific multi-line comment syntax, you can use consecutive single-line comments or triple-quoted strings (usually `”””` or `”’`) as a workaround. Triple quotes are technically multi-line strings but are often used for longer comments.
Why Bother with Python Comments ?
Let’s get real—comments are like the GPS for navigating your code. Without them, you might find yourself lost in a maze of logic and loops. Here’s why comments are indispensable:
1. Clarity :
Comments explain what the code is doing. They can describe the purpose of complex algorithms or remind you why you made a particular choice.
# Adding two numbers and returning the result
def add_numbers(a,b)
return a+b
2. Maintenance :
As your code grows, comments help you remember why you did something a certain way. They’re your past-self’s gift to your future-self.
3. Collaboration :
When working in teams, comments ensure everyone is on the same page. They can make the difference between a well-oiled team and a group of confused code wranglers.
Examples of Python Comments:
To make this journey through comments a bit more enjoyable, let’s sprinkle in some humor. Here’s a script where Python comments take on a life of their own!
# Debugging time : Watch out for bugs!
fixed_code=fix_bug("This code has a bug.")
print(fixed_code) # Expecting : This code has a feature
# Comments can be your best friend . They keep you sane!
# But remember, too many comments can be like having a chatty parrot that never shuts up
# So be wise , and use comments to enhance your code:
# 1. To explain the "why" behind your code
# 2. To mark TODOs and FIX ME reminders.
# 3. To provide a bit of comic relief in otherwise serious code
# And now , go forth and comment wisely , brave coder !
Comments in Python are like the quiet helpers that make coding easier for everyone. They don’t change how the program runs, but they play an important role in making the code clear, easy to read, and simple to understand. Think of them as helpful notes that explain what the code is doing and why certain choices were made. Whether you’re working alone or as part of a team, comments can save time, reduce confusion, and make your code much more manageable in the future.
Imagine coming back to your own project after months or even years. Without comments, it might feel like reading a foreign language! But with clear, well-written comments, everything makes sense again, and you can quickly pick up where you left off. Similarly, if you’re working with others, good comments ensure that everyone understands the purpose of the code, making teamwork much smoother.
Comments can also add personality to your code. While their main job is to explain things, they can sometimes be fun too! Programmers often leave clever notes, jokes, or friendly reminders in their comments, turning them into a creative part of coding.
By taking the time to write useful comments, you turn your code into more than just a set of instructions—it becomes a well-documented guide that anyone, including your future self, can easily follow. So the next time you start coding, don’t just focus on writing functional code—make it readable, understandable, and even enjoyable with great comments. Happy coding and happy commenting!