The Ultimate Guide to Python Tuples: Understanding Their Power and Practicality
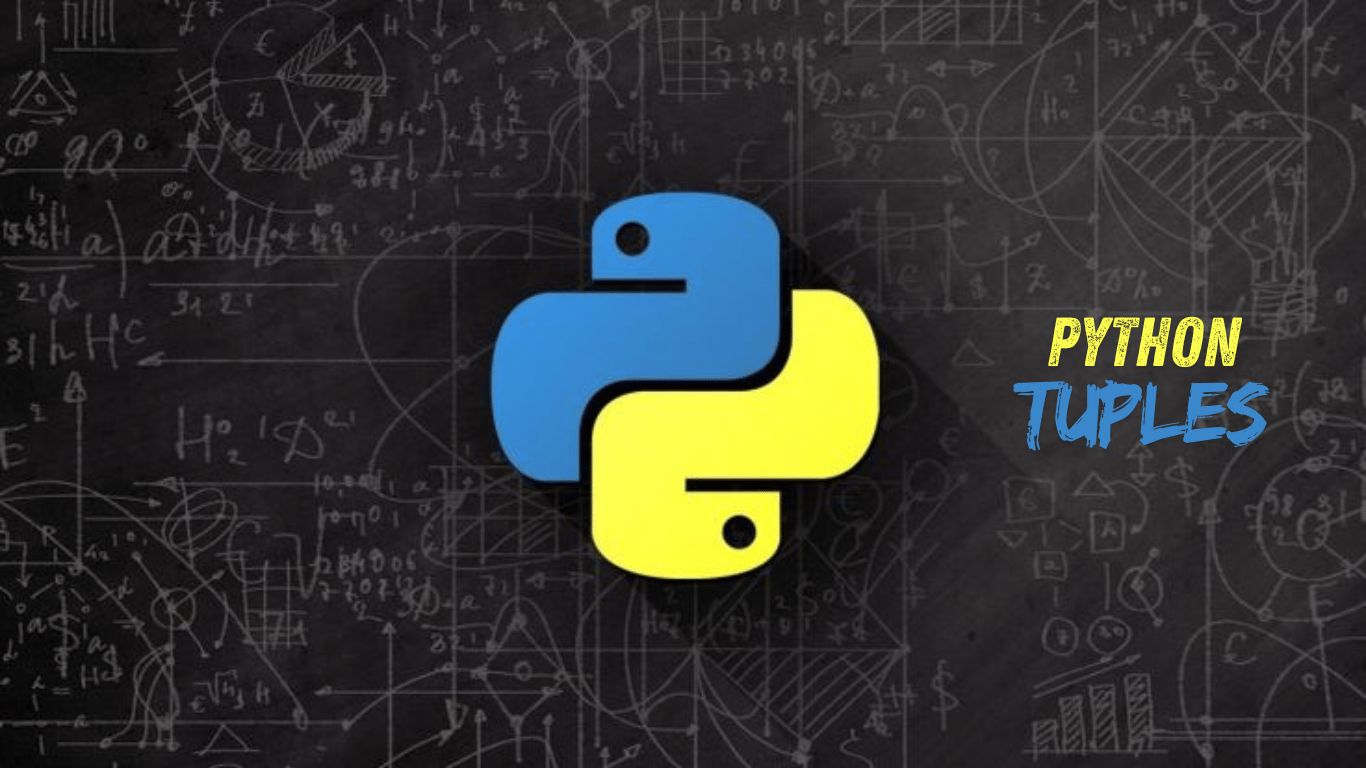
In the world of programming, there are moments that redefine your understanding of concepts, especially when it comes to data structures. You may find yourself learning about various ways to store and manipulate data, but have you ever paused to consider the power of Python tuples? Initially, they may seem like a simple concept, but once you delve deeper, you will realize their unique advantages and applications in your coding endeavors. This guide will equip you with everything you need to know about Python tuples, ensuring you appreciate their role in your programming toolkit.
Table of Contents
What is a Python Tuple?
A Python tuple is an immutable sequence type, which means that once you create a tuple, you cannot modify its contents. You define a tuple using parentheses, like so:
my_tuple = (1, 2, 3)
Tuples are ordered collections, meaning that the items you include in a tuple maintain their sequence. This characteristic can be particularly useful when you need to keep track of the order of data.
Why Use Tuples?
You might wonder why you would choose a tuple over a list, which is another popular data structure in Python. Here are a few reasons:
- Performance: Tuples generally require less memory and are faster to access than lists due to their immutability. This makes them a preferred choice in situations where performance is critical.
- Hashability: Because tuples are immutable, they can be used as keys in dictionaries, while lists cannot. This feature allows for more efficient data retrieval in certain applications.
- Data Integrity: When you want to ensure that your data remains unchanged throughout your program, tuples are an excellent option.
The Difference Between List and Tuple in Python
Understanding the differences between lists and tuples is crucial for making informed decisions about which data structure to use in your Python projects. Let’s break it down in the following comparison table:
Feature | List | Tuple |
---|---|---|
Mutability | Mutable | Immutable |
Syntax | Defined with brackets [] | Defined with parentheses () |
Performance | Slower | Faster |
Use Case | When data needs modification | When data integrity is key |
Key Differences
- Mutability: Lists can be modified after creation, while tuples cannot. If you need to add, remove, or change elements, lists are the way to go. However, if your data should remain constant, opt for tuples.
- Performance: Tuples generally consume less memory and allow for faster performance compared to lists. If you’re working with a large dataset, using tuples can improve efficiency.
- Use Case: Lists are suitable for collections that may change, while tuples are better for fixed collections of items. If you’re storing data that won’t change, such as coordinates or fixed settings, consider using tuples.
Key Features of Python Tuples
Now that you understand what tuples are and how they differ from lists, let’s explore some key features that make tuples a valuable data structure.
Immutability
One of the defining characteristics of tuples is their immutability. Once you create a tuple, you cannot alter its contents. This feature ensures that your data remains safe from unintended changes, which can be particularly useful in larger applications where data integrity is essential.
Ordered Nature
Tuples maintain the order of their elements. This means that when you create a tuple, the first element will always be at index 0, the second at index 1, and so forth. This ordered structure allows you to easily access items by their position.
Heterogeneous Elements
Tuples can store a collection of items that may vary in type. You can include integers, strings, lists, or even other tuples within a single tuple. For instance:
my_tuple = (1, "Hello", [1, 2, 3], (4, 5))
This flexibility allows you to create complex data structures without needing to use multiple lists or dictionaries.
Tuple Methods in Python
Despite being simpler than lists, tuples do have a couple of methods you might find useful. Here are the most common tuple methods:
1. count()
This method counts the occurrences of a specified value within a tuple. Here’s how it works:
my_tuple = (1, 2, 2, 3)
count_of_twos = my_tuple.count(2) # Returns 2
2. index()
The index()
method returns the index of the first occurrence of a specified value. If the value does not exist in the tuple, it raises a ValueError
. Here’s an example:
my_tuple = (1, 2, 3)
index_of_two = my_tuple.index(2) # Returns 1
By using these methods, you can effectively analyze and interact with your tuple data.
Python Tuple Example
Let’s look at some practical examples that showcase how to work with tuples in Python. Understanding how to create and manipulate tuples is crucial for your programming success.
Creating a Tuple
You can create a tuple by simply defining it with parentheses:
my_tuple = (10, 20, 30)
print(my_tuple) # Output: (10, 20, 30)
Accessing Tuple Elements
You can access elements in a tuple using indexing:
print(my_tuple[1]) # Output: 20
Iterating Through a Tuple
To iterate through a tuple, you can use a for loop:
for item in my_tuple:
print(item)
Tuple with Mixed Data Types
Tuples can contain different data types, making them versatile for various applications:
mixed_tuple = (42, "Hello", 3.14, True)
print(mixed_tuple)
Nested Tuples
You can also create nested tuples, which can be helpful for organizing related data:
nested_tuple = ((1, 2), (3, 4))
print(nested_tuple[1][0]) # Output: 3
By familiarizing yourself with these examples, you can begin to leverage tuples effectively in your own projects.
Common Questions about Python Tuples
In this section, we’ll address some frequently asked questions about Python tuples to provide additional clarity on this topic.
What is a Python tuple?
A Python tuple is an immutable data structure that holds a collection of items. Tuples are defined using parentheses and maintain the order of elements.
What is the difference between a list and a tuple in Python?
The primary differences between lists and tuples are mutability and syntax. Lists are mutable (changeable) and defined with brackets, while tuples are immutable (unchangeable) and defined with parentheses.
Can you modify a Python tuple?
No, you cannot modify a tuple after it has been created. However, you can create a new tuple by concatenating or slicing existing tuples.
How do you create a tuple in Python?
To create a tuple, simply enclose your items in parentheses, separating them with commas. For example:
my_tuple = (1, 2, 3)
Which of the following is a Python tuple?
A tuple is defined using parentheses. For example, (1, 2, 3)
is a tuple, while [1, 2, 3]
is a list.
Conclusion
Understanding Python tuples is an essential skill for any programmer. Their immutability, efficiency, and ability to hold mixed data types make them a powerful tool in your coding toolkit. Whether you’re using tuples to store constant data or as keys in a dictionary, their unique features offer numerous advantages in various programming scenarios.
Now that you have a comprehensive understanding of Python tuples, it’s time to put this knowledge into practice. Experiment with creating your own tuples, utilizing their methods, and discovering how they can enhance your projects. As you grow in your programming journey, embrace the potential of tuples and watch how they can streamline your coding experience.
If you found this guide helpful, don’t hesitate to share it with fellow developers or leave a comment below with your thoughts and experiences using Python tuples. Your engagement helps create a vibrant community where we can all learn and grow together!