Arrays in C: A Complete Guide to Arrays in C Programming
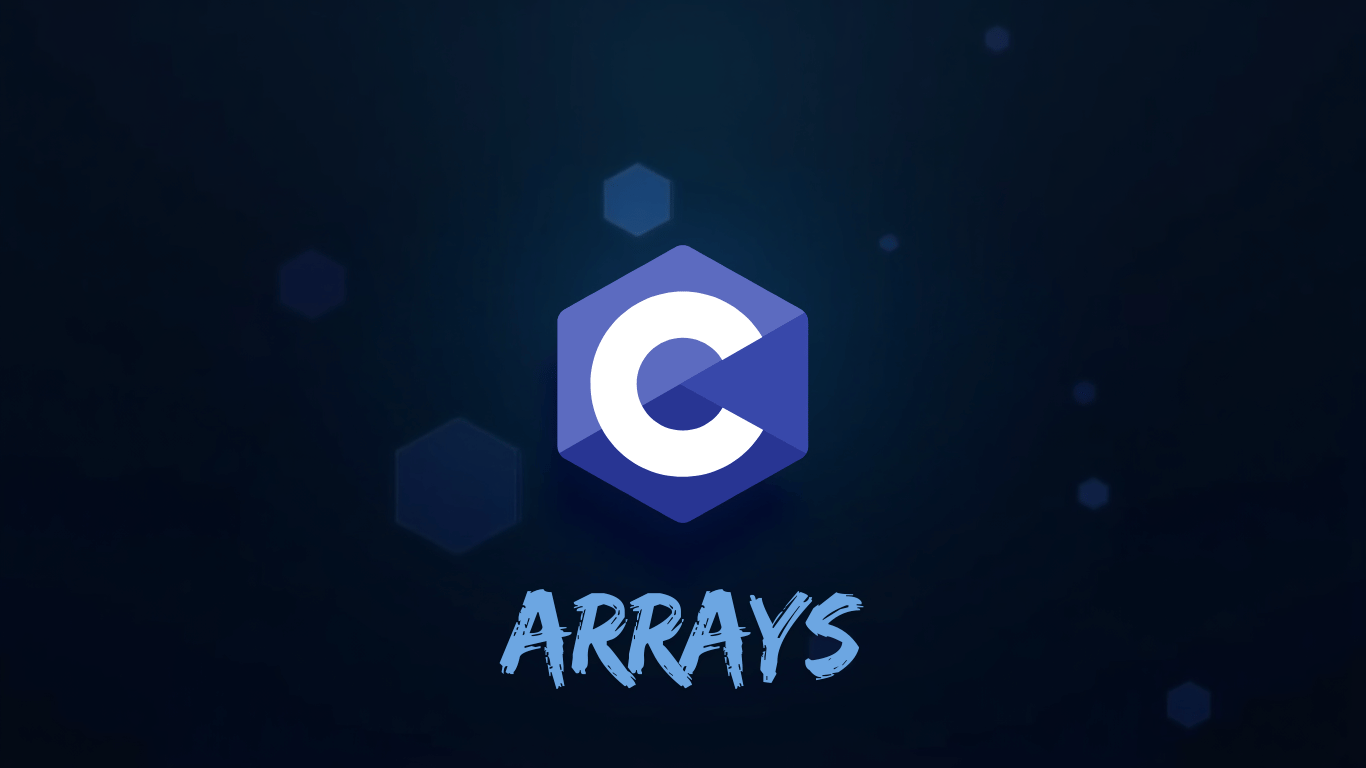
Introduction
Imagine you’re working on a project where you need to manage a collection of data efficiently. Instead of declaring multiple variables for each value, wouldn’t it be easier to store them all under one name? This is exactly where arrays in C programming shine. Arrays help you structure your data, optimize performance, and streamline your coding process. Whether you’re just starting with what is an array in C language, or looking to master concepts like array of pointers in C and sorting arrays in C, this guide has got you covered.
Table of Contents
What is an Array in C Language?
An array in C is a structured way to store multiple values of the same data type in contiguous memory locations. Arrays provide a powerful tool to handle bulk data efficiently, reducing redundancy and enhancing code performance.
Key Features of Arrays in C
- Stores multiple elements of the same type
- Fixed size, defined at declaration
- Uses contiguous memory allocation
- Accessed using an index
Syntax of Arrays in C
data_type array_name[size];
Example:
int numbers[5] = {1, 2, 3, 4, 5};
Arrays allow easy retrieval of elements using indices:
printf("%d", numbers[2]); // Output: 3
Types of Arrays in C
One Dimensional Array in C
A one-dimensional array is a linear collection of elements, where each element is accessed using an index.
Example:
int marks[5] = {90, 85, 88, 92, 75};
Accessing Elements:
printf("%d", marks[2]); // Output: 88
Multi-Dimensional Arrays in C
A multi-dimensional array consists of rows and columns, making it useful for storing tabular data.
Example of a 2D Array:
int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}};
Accessing Elements:
printf("%d", matrix[1][2]); // Output: 6
Three-Dimensional Arrays
In some cases, data needs to be stored in three dimensions, such as in graphical processing or simulations.
Example:
int cube[2][2][2] = { {{1,2}, {3,4}}, {{5,6}, {7,8}} };
Accessing Elements:
printf("%d", cube[1][0][1]); // Output: 6
Array of Pointers in C
An array of pointers is a collection of memory addresses pointing to different data elements.
Example:
char *names[] = {"Alice", "Bob", "Charlie"};
Benefits:
- Saves memory by referencing instead of copying data
- Allows flexible handling of dynamic data
- Helps in efficient string manipulation
Sorting Arrays in C
Sorting is essential for organizing data. Here are some common sorting techniques:
Bubble Sort
Bubble sort compares adjacent elements and swaps them if necessary to arrange them in order.
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
Quick Sort
Quick sort uses a divide-and-conquer approach for efficient sorting.
void quickSort(int arr[], int low, int high) {
// Sorting logic
}
Merge Sort
Merge Sort is another efficient sorting algorithm that divides the array into halves and merges them back in sorted order.
void mergeSort(int arr[], int l, int r) {
// Sorting logic
}
Sorting Algorithm | Best Case | Worst Case | Average Case |
---|---|---|---|
Bubble Sort | O(n) | O(n^2) | O(n^2) |
Quick Sort | O(n log n) | O(n^2) | O(n log n) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
Advantages & Disadvantages of Arrays
Advantages
- Efficient data storage and retrieval
- Simplifies handling large datasets
- Offers predictable memory access patterns
- Supports various sorting and searching algorithms
Disadvantages
- Fixed size may lead to wasted or insufficient memory allocation
- Insertion and deletion are inefficient compared to linked lists
- Requires contiguous memory, which may be a constraint in large applications
FAQ: Array in C
What is an array in C programming?
An array in C is a collection of elements of the same type stored in contiguous memory locations for efficient data management.
How do you declare an array in C?
You declare an array using the syntax: data_type array_name[size];
What is an array of pointers in C?
It is an array that stores addresses of other variables, allowing efficient memory management and flexible data handling.
How can you sort an array in C?
Sorting can be performed using algorithms like Bubble Sort, Quick Sort, and Merge Sort.
What is a multi-dimensional array in C?
A multi-dimensional array is an array of arrays, used for storing tabular or multi-level data, such as matrices and 3D structures.
Can I change the size of an array once declared?
No, the size of an array in C is fixed upon declaration. For dynamic resizing, you can use dynamically allocated arrays with malloc()
or calloc()
.
What is the difference between an array and a pointer in C?
An array is a collection of elements stored in contiguous memory, whereas a pointer stores the address of a variable and can dynamically point to different memory locations.
Conclusion
Mastering arrays in C allows you to optimize data handling, improve code efficiency, and streamline complex computations. Whether you’re working with one-dimensional arrays, arrays of pointers, or implementing sorting techniques, understanding arrays is essential. You can use arrays to manage structured data, store collections of values efficiently, and apply powerful algorithms to process your information effectively.
Now that you’ve learned the fundamentals of arrays in C, apply them in real-world projects. Experiment with different sorting techniques and explore advanced topics like dynamic memory allocation. The more you practice, the better your understanding will be. Take your programming to the next level and start coding today! Happy coding!