JavaScript : Advanced Functions
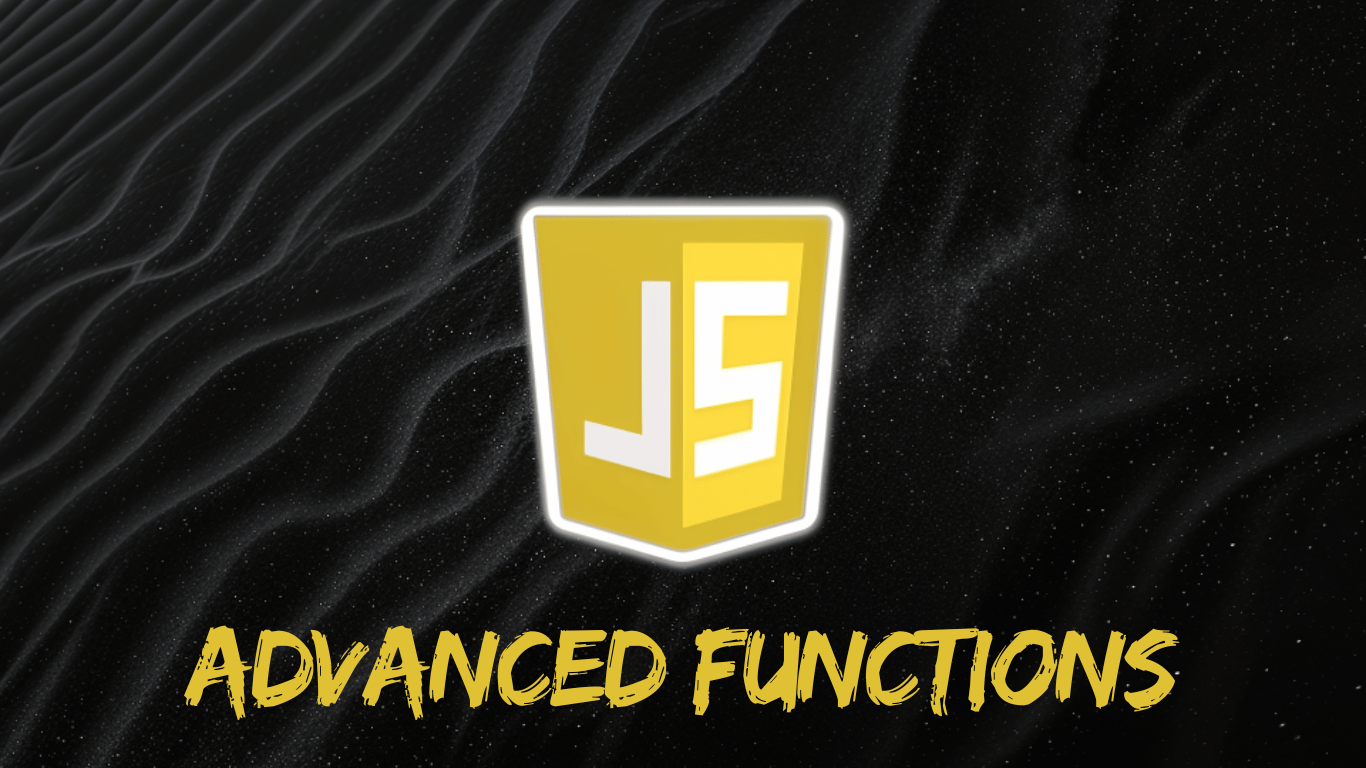
As you continue to evolve as a JavaScript developer, mastering more advanced techniques will significantly elevate the quality of your code. Whether you’re creating more efficient web applications, simplifying complex tasks, or improving the readability and maintainability of your projects, advanced JavaScript functions offer the tools to get there.
In this guide, you’ll explore key advanced functions in JavaScript—like arrow functions, closures, and higher-order functions—that can help you streamline your development process. By the end, you’ll not only understand these concepts but also be able to apply them to solve real-world coding problems effectively.
Table of Contents
What Is a JavaScript Function?
Before diving into advanced concepts, let’s quickly revisit the foundation: functions in JavaScript. A function is a reusable block of code designed to perform a specific task. When working with functions, you can pass parameters, return values, and organize code more modularly. This flexibility is what makes JavaScript so powerful.
However, once you have a solid grasp of basic functions, you’ll soon realize that JavaScript has a whole new world of advanced functions waiting for you to unlock. From closures to arrow functions and everything in between, mastering these concepts will take your code to the next level.
Advanced JavaScript Functions: What’s Inside?
In this section, we’ll explore a range of advanced JavaScript function techniques that will help you write cleaner, more efficient, and scalable code. These include closures, higher-order functions, the powerful map and filter functions, and much more. Let’s dive into the details.
1. Closures: Access Variables Beyond Their Scope
Closures are one of JavaScript’s most powerful features. At its core, a closure is a function that “remembers” its outer environment, even after the parent function has finished execution. This allows you to maintain access to variables from the parent function, making closures incredibly useful for scenarios like private variables or managing state in an app.
Key Points About Closures:
- Closures allow inner functions to access variables from their parent scope.
- They’re useful for managing private data, creating functions with persistent states, or even implementing callback functions.
Example:
function outer() {
let counter = 0;
return function inner() {
counter++;
console.log(counter);
}
}
const increment = outer();
increment(); // Output: 1
increment(); // Output: 2
In the above example, the inner() function is a closure because it has access to the counter variable even after outer() has completed execution.
2. Higher-Order Functions: Functions That Work with Other Functions
A higher-order function is any function that takes one or more functions as arguments or returns a function as a result. These functions allow you to treat other functions as values, enabling dynamic behavior in your code.
Common Higher-Order Functions:
- Map Function JavaScript: Transforms elements of an array.
- Filter Function JavaScript: Filters out elements based on a condition.
- Reduce Function: Combines elements of an array into a single result.
Example Using the map and filter Functions:
const numbers = [1, 2, 3, 4, 5];
// Using map to double the numbers
const doubled = numbers.map(num => num * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
// Using filter to get even numbers
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
These higher-order functions allow you to process arrays in a clean, declarative manner. The map() function transforms each element of an array, while filter() keeps only the elements that meet the specified condition.
3. Arrow Functions: Simplified Syntax with Potential Pitfalls
Arrow functions (introduced in ES6) provide a more concise way to write functions. They simplify syntax, but there’s a key difference from traditional function expressions—arrow functions do not have their own this context. This can sometimes lead to unexpected behavior if not used carefully.
Key Benefits of Arrow Functions:
- More concise syntax.
- No need to bind this in many cases.
Example of Arrow Function:
const add = (a, b) => a + b;
console.log(add(5, 3)); // Output: 8
Arrow functions are ideal for simple functions, such as those used in the map() , filter(), or reduce() methods, as they make your code cleaner and more readable.
4. The this Keyword: Understanding Context
In JavaScript, the value of this is dynamically determined by how a function is called. In advanced functions, understanding how this works can help avoid tricky situations, especially when using higher-order functions like map() , filter() , or in object-oriented programming.
Example of this Context:
const person = {
name: 'John',
greet() {
console.log(`Hello, ${this.name}`);
}
};
person.greet(); // Output: Hello, John
However, when using arrow functions, this behaves differently. It takes the this value from the surrounding context, which is often useful when working with functions like map() or filter() .
5. The call , apply , and bind Methods: Borrowing Functionality
These three method call()
, apply()
, and bind()
—allow you to explicitly set the this
value for a function call. While they all serve the same purpose, they differ in how arguments are passed.
Key Differences:
call()
: Executes the function immediately with a specifiedthis
value.apply()
: Similar tocall()
, but it takes arguments as an array.bind()
: Returns a new function, but doesn’t execute it immediately. You can invoke the function later.
Example:
const person = {
name: 'Alice',
greet() {
console.log(`Hello, ${this.name}`);
}
};
const anotherPerson = { name: 'Bob' };
person.greet.call(anotherPerson); // Output: Hello, Bob
In this example, the greet()
function is borrowed from person
but executed with the this
value of anotherPerson
using call()
.
6. Recursion: Simplifying Complex Problems
Recursion occurs when a function calls itself. It’s useful for tasks that have a repetitive or nested structure, such as tree traversal or calculating factorials. By calling itself, a recursive function can solve problems that might otherwise require loops or complicated conditionals.
Example of Recursion:
function factorial(n) {
if (n === 0) return 1;
return n * factorial(n - 1);
}
console.log(factorial(5)); // Output: 120
Recursion is an elegant solution to problems where you can break the task into smaller, more manageable sub-tasks.
7. The Spread Operator: Unpacking Arrays and Objects
The spread operator (...
) allows you to unpack elements from arrays or objects and pass them as individual arguments in a function call. It simplifies working with arrays and objects, making your code cleaner and more efficient.
Example of Using the Spread Operator:
const nums = [1, 2, 3];
const newNums = [...nums, 4, 5];
console.log(newNums); // Output: [1, 2, 3, 4, 5]
The spread operator is highly versatile, allowing you to merge arrays, create copies of objects, or expand arguments when invoking functions.
Conclusion: Unlocking New Possibilities with Advanced JavaScript Functions
By mastering advanced JavaScript functions like closures, higher-order functions, arrow functions, and recursion, you’re equipping yourself with the tools to build more efficient, flexible, and powerful web applications. With these skills in your toolkit, you’ll be able to write cleaner, more modular code that can easily be extended and maintained.
Now it’s your turn to put these concepts into action! Start incorporating advanced JavaScript functions into your projects today, and watch your development process become smoother and more enjoyable. Want to learn more about the latest JavaScript tricks and techniques? Stay tuned for more updates and resources to keep your coding skills sharp in 2025 and beyond.
Ready to level up your JavaScript game? Start experimenting with closures, higher-order functions, and arrow functions in your next project, and see how they transform your code!