Logical Operators in C: A Complete Guide with Examples
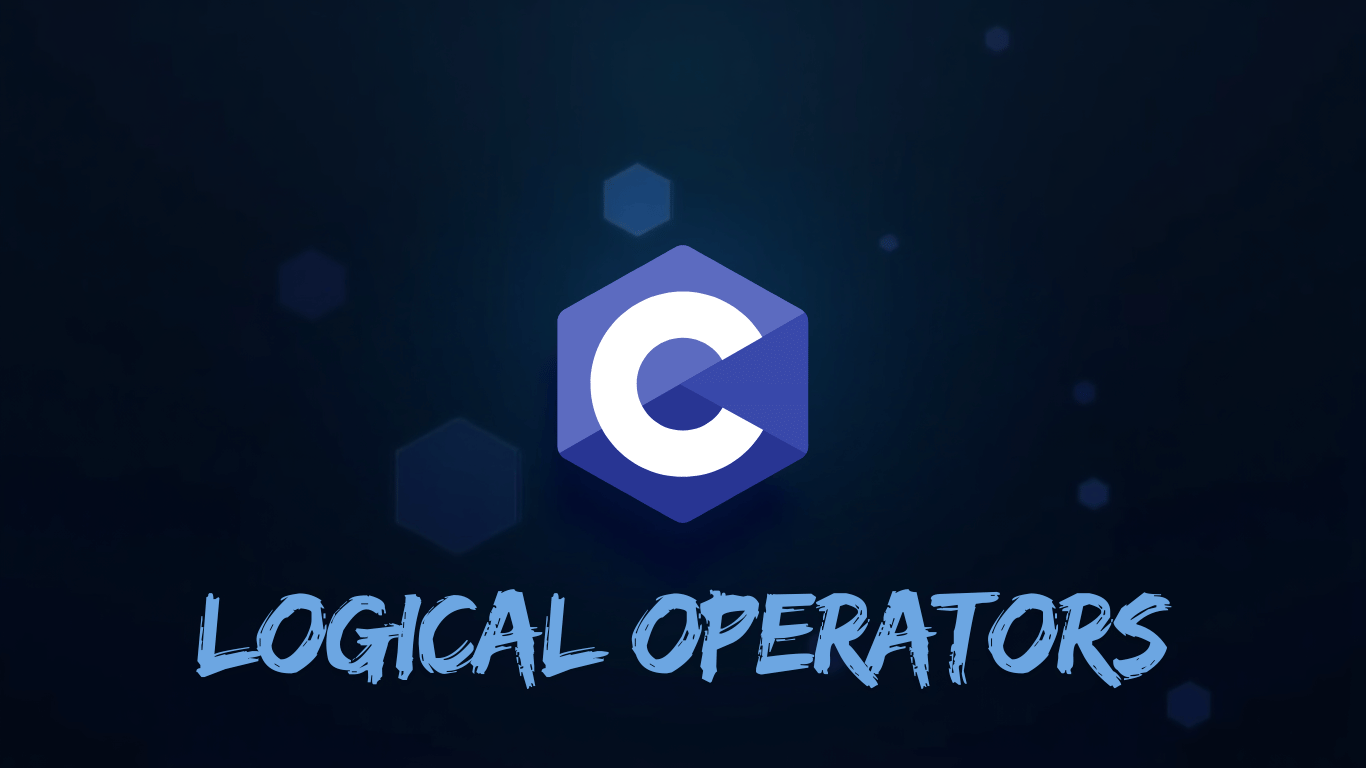
Introduction: Why Logical Operators in C Matter
When coding in C, you frequently need to make decisions based on multiple conditions. Imagine you’re writing a program that checks if a user is both logged in and has the necessary permissions before granting access. This is where logical operators in C come into play, allowing you to evaluate multiple conditions efficiently.
In this guide, you’ll learn about logical operators in C, their types, usage, and how they differ from bitwise logical operators in C. We’ll also explore practical applications and best practices to ensure you master them completely.
Table of Contents
What is a Logical Operator in C?
Logical operators in C help you make decisions based on multiple conditions. They return true (1) or false (0) depending on whether the given conditions hold. These operators are essential in control flow statements such as if , while , and for loops.
Use Cases of Logical Operators in C
- Checking multiple conditions in an
if
statement. - Controlling loop execution based on logical conditions.
- Implementing authentication systems.
- Optimizing game logic and AI-based decisions.
Let’s break down the different logical operators in C.
Types of Logical Operators in C with Examples
Logical AND Operator (&&
)
- Functionality: Returns true if both conditions are true; otherwise, returns false.
- Example Code:
#include <stdio.h>
int main() {
int a = 10, b = 20;
if (a > 5 && b > 15) {
printf("Both conditions are true\n");
}
return 0; }
- Truth Table: Condition 1 Condition 2 Result (
&&
) 0 0 0 0 1 0 1 0 0 1 1 1
Logical OR Operator (||
)
- Functionality: Returns
true
if at least one condition is true. - Example Code:
int age = 18;
if (age < 16 || age > 60) {
printf("You are not eligible\n");
}
- Truth Table: | Condition 1 | Condition 2 | Result (
||
) | |————|————|————–| | 0 | 0 | 0 | | 0 | 1 | 1 | | 1 | 0 | 1 | | 1 | 1 | 1 |
Logical NOT Operator (!
)
- Functionality: Negates the given condition.
- Example Code:
int flag = 0;
if (!flag) {
printf("Flag is false\n");
}
- Truth Table: Condition Result (
!Condition
) 0 1 1 0
Bitwise Logical Operators in C
Difference Between Logical and Bitwise Logical Operators
- Logical Operators (
&&
,||
,!
): Used for boolean logic. - Bitwise Operators (
&
,|
,^
,~
): Work at the bit level.
Examples of Bitwise Logical Operators
- Bitwise AND (
&
):5 & 3
results in1
(0101 & 0011 = 0001) - Bitwise OR (
|
):5 | 3
results in7
(0101 | 0011 = 0111) - Bitwise XOR (
^
):5 ^ 3
results in6
(0101 ^ 0011 = 0110) - Bitwise NOT (
~
):~5
results in-6
(in two’s complement representation)
Example Code:
#include <stdio.h>
int main() {
int x = 5, y = 3;
printf("Bitwise AND: %d\n", x & y);
printf("Bitwise OR: %d\n", x | y);
return 0;
}
Practical Examples of Logical Operators in C
Example 1: User Authentication System
char username[] = "admin";
char password[] = "1234";
if (strcmp(inputUser, username) == 0 && strcmp(inputPass, password) == 0) {
printf("Login Successful\n");
}
Example 2: Traffic Light System
if (light == "red" || pedestrianCrossing == 1) {
printf("Stop\n");
}
Example 3: AI Decision-Making in a Game
if (playerHealth > 50 && enemyDistance < 20) {
attack();
}
Common Mistakes and Best Practices
Common Mistakes
- Using
=
instead of==
in conditions. - Misusing
!
(logical NOT) with non-boolean values. - Confusing
&&
with&
.
Best Practices
- Use Parentheses to enhance readability.
- Avoid Redundant Conditions to optimize performance.
- Understand Operator Precedence to avoid unexpected results.
FAQ – Logical Operators in C
What is a logical operator in C?
Logical operators help perform boolean operations on conditions, returning true
or false
.
What is the difference between logical and bitwise operators in C?
Logical operators work with boolean values, while bitwise operators manipulate individual bits.
Can we use logical operators in loops?
Yes, they are used in while
, for
, and do-while
loops for condition evaluation.
What is the output of !0
and !1
in C?
!0
gives1
.!1
gives0
.
Conclusion: Master Logical Operators in C with Practice
Logical operators in C are fundamental to making decisions in programming. By mastering them, you improve your ability to write efficient and error-free code. Start by experimenting with simple conditions and gradually move on to complex logic. Want to learn more about C programming? Keep exploring!
Are you struggling with C programming concepts? Drop your questions in the comments or explore more tutorials on our website!