Step by Step Guide : Manipulate Strings in JavaScript
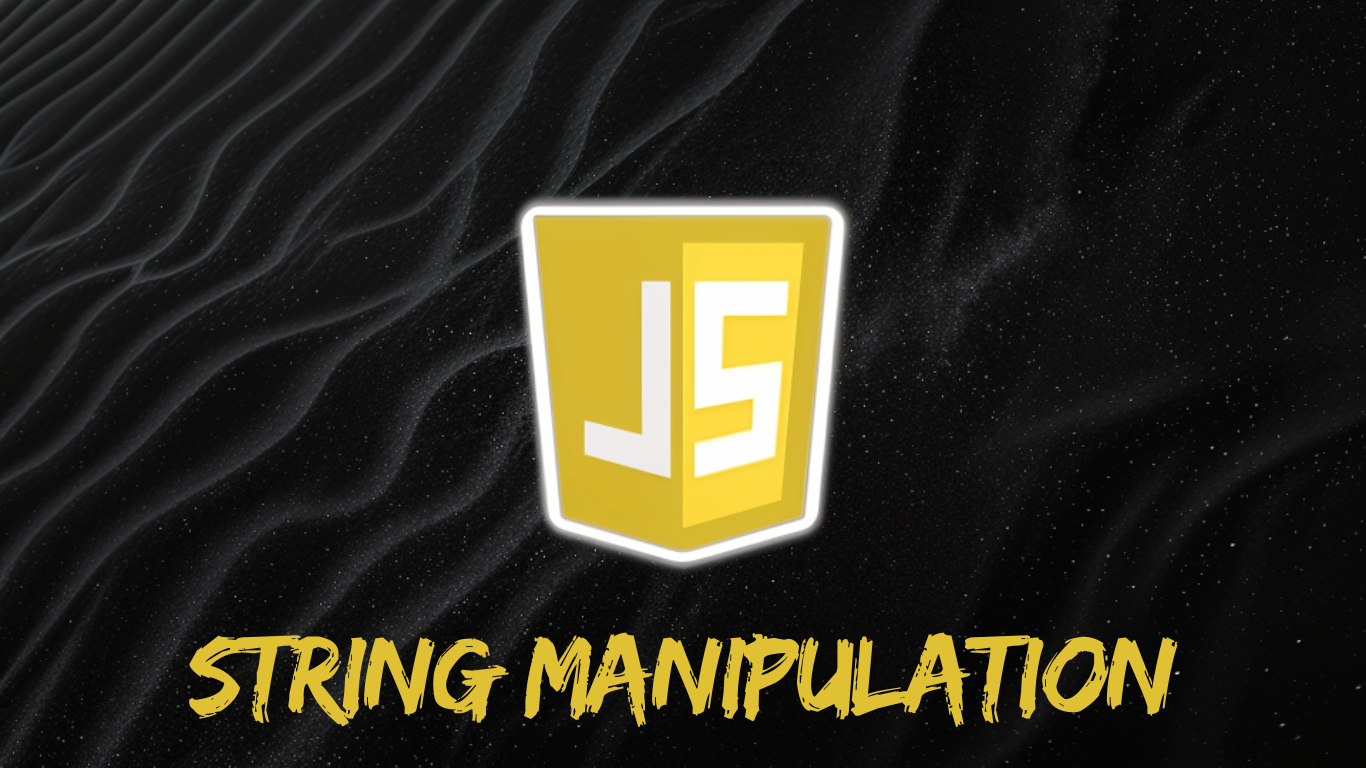
Manipulating Strings is an essential skill for developers, as it allows them to effectively handle and modify data within their applications. Whether you’re working with user input, processing data files, or displaying information on a webpage, knowing how to extract, modify, and format text is key to building dynamic and interactive features.
In this guide, we explore a variety of methods that enable you to perform different operations on data. From basic tasks, such as pulling out specific sections , to more advanced techniques like using regular expressions for pattern matching and replacement, you’ll learn how to manipulate strings in a way that is both efficient and flexible. Mastering these tools will empower you to handle a wide range of related tasks in your projects with ease.
Table of Contents
Extracting and Manipulating Strings :
Extracting Characters :
There are different ways to extract a character from a string in JS. Among the available methods , charAt(), at(), and charCodeAt() are commonly used.
– charAt(index): This method takes an index as a parameter and returns the character at that position. If the index is beyond the string’s length, it returns an empty string.
– at(index): Introduced in ES2022, this method is similar to charAt() but returns undefined if the index is out of range. A key advantage is that it supports negative indexing, allowing access to characters from the end.
– charCodeAt(index): Unlike the previous methods, charCodeAt() returns the UTF-16 code of the character at the specified index. It’s useful when numeric character codes are needed.
Extracting Substrings :
– charAt(index): This method takes an index as a parameter and returns the character at that position. If the index is beyond the length, it returns an empty string.
– substring(start, end): This method extracts a part of the text from the start index to the end index (non-inclusive). If the end index is not provided, it extracts until the end.
– slice(start, end): Similar to substring(), but slice() also supports negative indexing, which allows specifying positions from the end.
Converting Letter Cases :
Converting to uppercase or lowercase is simple using toUpperCase() and toLowerCase().
– toUpperCase(): This method converts the entire string to uppercase letters.
– toLowerCase(): This method converts all characters in a string to lowercase.
Modifying and Formatting Strings :
Concatenating Strings :
When handling input from external sources, white spaces can be an issue. JavaScript provides three methods to handle this:
– Using the + operator to concatenate strings.
– Using the concat() method, which joins two or more strings.
– Leveraging template literals to easily combine strings and include expressions.
Trimming White Spaces :
When handling input from external sources, white spaces can be an issue. JavaScript provides three methods to handle this:
– trimStart() removes white spaces from the beginning of a string.
– trimEnd() removes white spaces from the end of the text.
– trim() removes white spaces from both ends.
Adding Padding :
To add characters to the start or end of a string, padStart() and padEnd() can be used.
– padStart(length, substring): Adds the specified substring to the beginning until it reaches the desired length.
– padEnd(length, substring): Adds the specified substring to the end until the target length is reached.
Repeating Strings :
The repeat() method allows creating a new text by repeating the original a specified number of times.
Splitting and Searching Strings :
Splitting Strings into arrays :
Strings can be split into arrays using the split() method. This method divides a text into an array of substrings based on a specified separator.
Searching within strings :
Several methods are available for searching within strings:
– indexOf() and lastIndexOf(): Both methods search for a substring and return its index. The former returns the first occurrence, while the latter returns the last.
– includes(): This method checks whether a substring exists within a string and returns true or false.
– startsWith() and endsWith(): These methods check if a string begins or ends with a specific substring.
Advanced Search with Regular expressions :
For more complex searching, we can use regular expressions (Regex), combined with various methods.
– search(): This method is similar to indexOf() but accepts a regular expression, returning the index of the first match.
– match() and matchAll(): These methods return an array of matches based on the provided regular expression. With the global flag, match() returns all matches, while matchAll() returns an iterable object containing detailed match information.
Replacing Patterns in Strings :
When it comes to replacing specific patterns in text, the replace()
method is an incredibly powerful tool. This method allows you to search for a particular sequence of characters or a pattern within the text and replace it with something new. What makes it even more useful is that you can either provide a direct substring that you want to replace, or you can use a regular expression to match more complex patterns.
For example, if you want to replace a word throughout a block of text, you can simply specify that word as the target, and it will be replaced everywhere it appears. Alternatively, by using regular expressions, you can perform more advanced replacements, such as changing patterns based on certain conditions, like replacing all instances of phone numbers or email addresses with a standardized format.
This method is highly flexible and can be used in a wide variety of situations, from making simple text corrections to performing complex transformations of your data. With the replace()
method, you have the power to customize how text is manipulated, making it an essential tool for many different tasks that involve text processing.
Conclusion :
In this guide, we’ve taken a deep dive into some of the most important techniques for working with text data. These techniques help you manipulate and modify text in ways that are useful for almost any project. We covered a range of basic operations like extracting specific characters, slicing text into smaller parts, and changing the case of letters.
You also learned how to join multiple pieces of text together, trim unwanted spaces, and add extra padding to your text. For situations where you need to repeat or split text into smaller chunks, we explored methods that make these tasks simple and efficient.
You also learned how to join multiple pieces of text together, trim unwanted spaces, and add extra padding. For situations where you need to repeat or split text into smaller chunks, we explored methods that make these tasks simple and efficient.
Mastering these text manipulation techniques will enable you to handle and process text effectively in any program you build. Whether you’re working with user input, processing data from files, or dealing with web content, these methods are your go-to tools for working with text.