Python Booleans Explained: Master Boolean Operators & Logic in Python
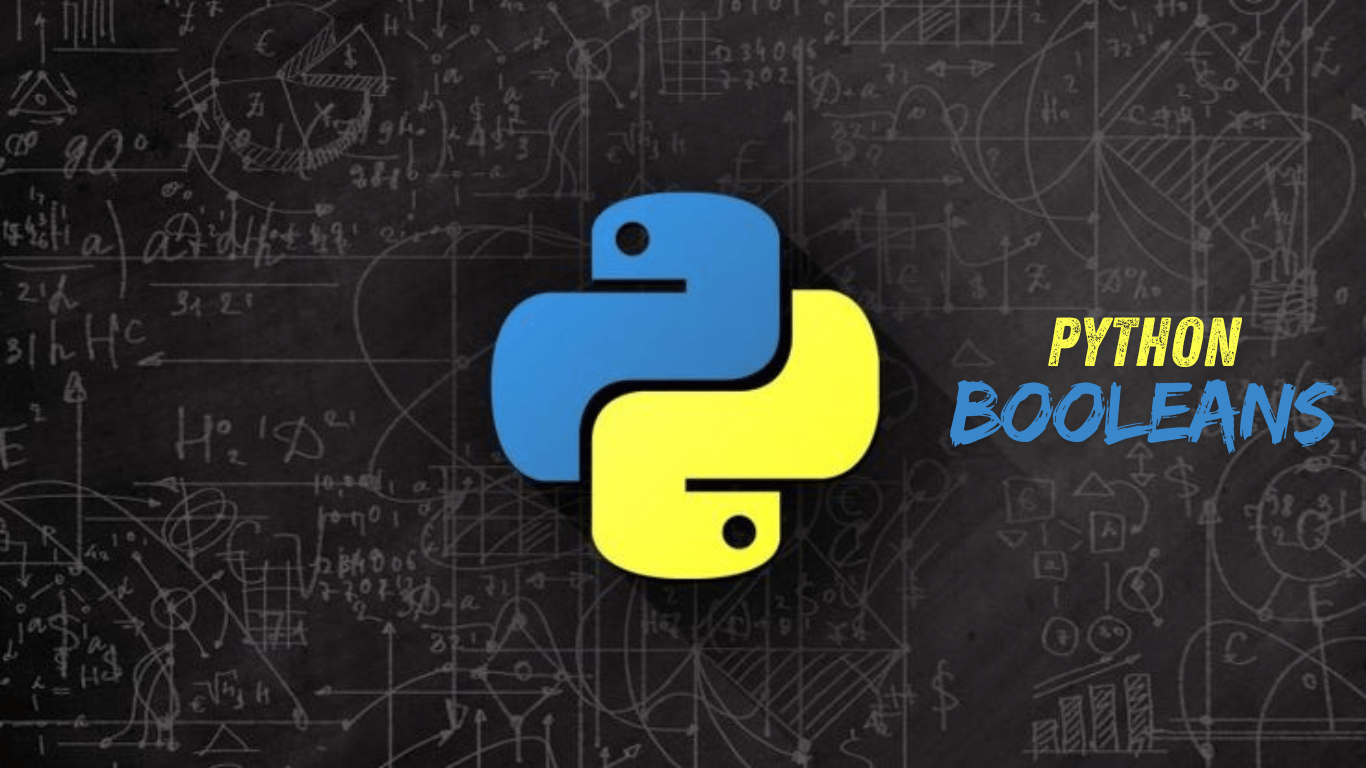
Introduction: Why Python Booleans Matter
Imagine making choices without clear answers—confusing, right? When writing Python code, Boolean values provide the “yes” or “no” needed to control logic and make decisions. Understanding Booleans is crucial because they underpin conditions, loops, and logic-based operations.
Whether you’re a beginner or an experienced coder, mastering Python Boolean will elevate your programming skills. In this guide, you’ll learn about Boolean values, operators, truthy and falsy values, and real-world applications.
Table of Contents
What Are Python Booleans?
Defining Booleans in Python
In Python , Boolean values belong to the bool data type and have only two possible states: True and False. These values help evaluate conditions in logical statements.
is_python_fun = True
print(is_python_fun) # Output: True
Python treats True as 1 and False as 0. This means Booleans can be used in mathematical operations:
print(True + True) # Output: 2
print(False + 1) # Output: 1
Python Boolean Operators: The Core of Logical Decision-Making
Understanding Boolean Operators in Python
Python provides three logical operators to work with Boolean values:
and
– ReturnsTrue
if both conditions areTrue
.or
– ReturnsTrue
if at least one condition isTrue
.not
– Reverses the Boolean value.
Boolean Operators Table
Operator | Description | Example | Result |
---|---|---|---|
and | Both must be True | True and False | False |
or | At least one must be True | True or False | True |
not | Reverses the value | not True | False |
Practical Examples of Boolean Operators
x = True
y = False
print(x and y) # Output: False
print(x or y) # Output: True
print(not x) # Output: False
Truthy and Falsy Values in Python
What Are Truthy and Falsy Values?
Python considers some values as True
or False
even if they are not explicitly True
or False
.
Falsy Values:
0
""
(empty string)[]
(empty list){}
(empty dictionary)None
False
Truthy Values:
- Any non-zero number
- Non-empty strings
- Lists, tuples, and dictionaries containing elements
Truthy and Falsy Values Table
Value | Evaluates To |
---|---|
0 | False |
"" | False |
None | False |
1 | True |
"Hello" | True |
[1, 2, 3] | True |
Boolean Expressions in Python Conditional Statements
Using Booleans in If Statements
Boolean expressions determine whether a block of code runs or not:
is_adult = True
if is_adult:
print("You can vote!")
Combining Boolean Expressions in Conditions
Use and , or , and not to combine conditions.
age = 20
if age > 18 and age < 65:
print("Eligible for work")
Python Boolean Functions & Built-in Methods
The bool() Function
Converts values into Boolean True
or False
:
print(bool(0)) # Output: False
print(bool(42)) # Output: True
Common Python Functions That Return Boolean Values
isinstance()
,startswith()
,endswith()
, etc.
print(isinstance(10, int)) # Output: True
Boolean Operators vs. Bitwise Operators
Key Differences Between Boolean and Bitwise Operators
- Boolean-Operators (
and
,or
,not
) work withTrue
andFalse
values. - Bitwise Operators (
&
,|
,^
) operate on binary values.
Boolean vs. Bitwise Operators Table
Operator | Type | Example | Output |
---|---|---|---|
and | Boolean | True and False | False |
& | Bitwise | 5 & 3 | 1 |
or | Boolean | True or False | True |
` | ` | Bitwise | `5 |
Advanced Boolean Concepts in Python
Short-Circuit Evaluation in Python
Python stops evaluating Boolean expressions as soon as the result is determined.
def check():
print("Function called")
return True
print(False and check()) # Output: False (Function is not called)
print(True or check()) # Output: True (Function is not called)
Boolean Operators in List Comprehensions
Use Booleans to filter lists.
numbers = [1, 2, 3, 4, 5]
evens = [num for num in numbers if num % 2 == 0]
print(evens) # Output: [2, 4]
Frequently Asked Questions (FAQ) on Python-Booleans
What is a Boolean in Python?
A Boolean is a data type with two values: True
and False
.
How do Boolean operators work in Python?
Boolean-operators (and
, or
, not
) control logical decisions by evaluating conditions.
What are truthy and falsy values in Python?
Truthy values evaluate to True
, while falsy values (like 0
, None
, ""
) evaluate to False
.
What is the difference between and
and &
in Python?
and is a Boolean operator, while &
is a bitwise operator used for binary operations.
Conclusion: Master Python Booleans for Smarter Coding
- Recap Key Takeaways: Boolean values guide program flow, simplify logic, and help in decision-making.
- Encouragement to Practice: Try writing Python scripts using Booleans in real-world scenarios.
- Engagement: Share your favorite Boolean tricks or questions in the comments!
By mastering Boolean logic, you’ll write cleaner, more efficient Python code. Keep experimenting and happy coding! 🚀