Mastering Python Lists: A Comprehensive Guide to Sorting, Slicing & More
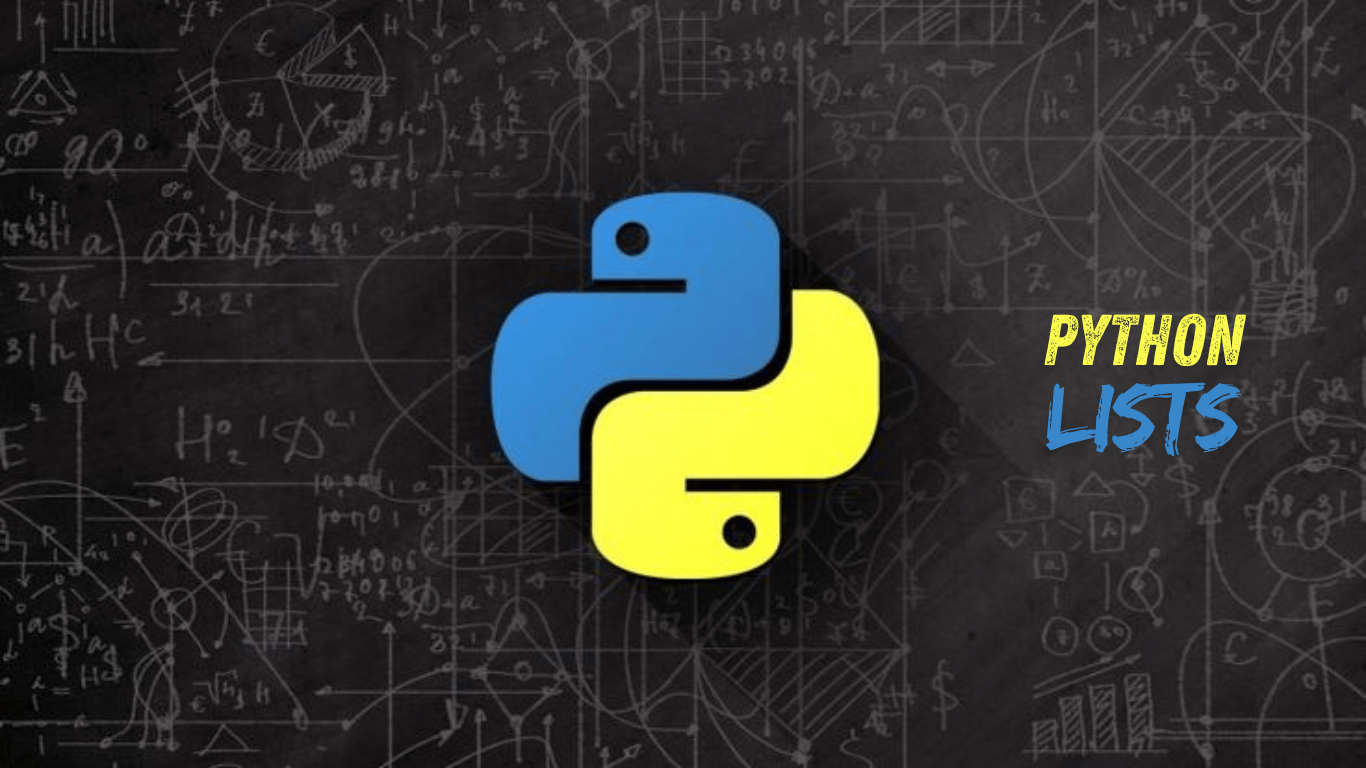
Introduction: Why Python Lists Matter in Your Coding Journey
Think back to the first time you dabbled in Python. You probably came across lists, one of the most versatile and essential data structures in the language. Whether you’re storing names, numbers, or objects, lists help you manage data efficiently. But are you truly leveraging their full potential?
In this guide, you’ll uncover the secrets behind Python lists, from sorting techniques to slicing strategies, and even explore alternatives like linked lists. By the end, you’ll have the knowledge to optimize your code and write cleaner, faster programs.
Table of Contents
What is a Python List?
A Python list is an ordered, mutable collection of items. Unlike arrays, which store only one data type, lists can hold a mix of integers, strings, and even other lists.
Key Features:
- Ordered: Maintains the sequence of elements.
- Mutable: Can be modified after creation.
- Supports Duplication: Identical elements can appear multiple times.
Example:
my_list = [1, "hello", 3.14, True]
print(my_list)
Creating & Manipulating Lists
Basic List Creation
- Using square brackets:
my_list = [1, 2, 3]
- Using list() constructor:
my_list = list((1, 2, 3))
Modifying Lists
- Adding Elements:
append()
,extend()
,insert()
- Removing Elements:
remove()
,pop()
,del
Python List Length: Measuring List Size
Use len()
to determine list size:
my_list = [10, 20, 30]
print(len(my_list)) # Output: 3
Python List Slicing: Extracting Subsets
Operation | Example | Result |
---|---|---|
Slice first 3 elements | my_list[:3] | [1, 2, 3] |
Slice from index 2 to 5 | my_list[2:5] | [3, 4, 5] |
Slice with step | my_list[::2] | [1, 3, 5] |
Reverse a list | my_list[::-1] | [5, 4, 3, 2, 1] |
Python Sort List: Sorting Techniques
Sorting Methods in Python
- Ascending order:
list.sort()
- Descending order:
list.sort(reverse=True)
- Sorted copy:
sorted(list)
Custom Sorting:
names = ["Alice", "Bob", "Eve"]
names.sort(key=len) # Sort by length
Linked List in Python: An Alternative to Lists?
What is a Linked List?
A linked list is a dynamic data structure where elements (nodes) are linked together.
Differences from Python Lists:
Feature | List | Linked List |
---|---|---|
Memory Allocation | Contiguous | Dynamic |
Indexing | Fast | Slower |
Insert/Delete | Slower | Faster |
Linked List Implementation:
class Node:
def __init__(self, data):
self.data = data
self.next = None
Difference Between List and Tuple in Python
Feature | List | Tuple |
---|---|---|
Mutability | Mutable | Immutable |
Performance | Slower | Faster |
Syntax | [1, 2, 3] | (1, 2, 3) |
Python List to String Conversion
Using join()
:
words = ["Python", "is", "awesome"]
sentence = " ".join(words)
print(sentence) # Output: Python is awesome
Common Python List Operations
- Check if an item exists:
if "apple" in my_list:
- Copying lists:
new_list = old_list.copy()
- Clearing lists:
list.clear()
- Merging lists:
list1 + list2
FAQs: Python Lists Explained
What is the difference between a list and an array in Python?
Lists support heterogeneous elements, while arrays (from NumPy) are optimized for numerical operations.
How do I remove duplicates from a list?
unique_list = list(set(my_list))
How can I shuffle a list randomly?
import random
random.shuffle(my_list)
Conclusion: Master Lists for Cleaner Code
Python lists are the backbone of efficient programming. With sorting, slicing, and conversion techniques, you can improve performance and readability. Try implementing these in your own projects today and enhance your coding expertise!